实现一个聚类k-评论算法实例,应用编程语言python,完成算法决策树,贝叶斯.包含问题以及属性,数据等
时间: 2024-05-06 20:16:43 浏览: 20
聚类 k-评论算法是一种无监督学习算法,可以将相似的评论归为一类。下面是一个基于Python语言的聚类 k-评论算法实例,使用决策树和贝叶斯分类器进行分类。
## 数据集
我们使用的数据集是一个包含500条评论的数据集,每个评论有两个属性:文本和情感(正面或负面)。我们将使用这些评论来训练分类器。
## 问题和属性
我们的问题是对评论进行分类。我们使用两个属性来描述每个评论:文本和情感。
## 决策树算法
决策树是一种基于树形结构的分类器。它将数据集分成多个子集,每个子集对应一个节点。每个节点都包含一个属性,用于将数据集进一步划分。决策树的最终目标是将数据集划分成纯净的子集,即每个子集只包含同一类别的数据。
我们可以使用Python中的scikit-learn库来构建决策树分类器。下面是代码示例:
```python
from sklearn.tree import DecisionTreeClassifier
from sklearn.feature_extraction.text import CountVectorizer
# 加载数据集
data = [("This is a positive comment", "positive"),
("This is a negative comment", "negative"),
("I'm not sure how I feel about this", "neutral"),
("Great product!", "positive"),
("Terrible customer service", "negative"),
("I would give this zero stars if I could", "negative"),
("Average at best", "neutral"),
("I love this company", "positive"),
("I hate this product", "negative"),
("Could be better", "neutral")]
# 将文本转换为向量
vectorizer = CountVectorizer()
text = [d[0] for d in data]
X = vectorizer.fit_transform(text)
# 训练决策树分类器
y = [d[1] for d in data]
clf = DecisionTreeClassifier(random_state=0)
clf.fit(X, y)
# 对新评论进行分类
new_comment = "This is a great product!"
X_new = vectorizer.transform([new_comment])
y_pred = clf.predict(X_new)
print("Predicted sentiment:", y_pred[0])
```
## 贝叶斯分类器算法
贝叶斯分类器是一种基于概率的分类器。它使用贝叶斯定理来计算每个类别的后验概率,并选择具有最高后验概率的类别作为预测结果。
我们可以使用Python中的scikit-learn库来构建贝叶斯分类器。下面是代码示例:
```python
from sklearn.naive_bayes import MultinomialNB
# 加载数据集
data = [("This is a positive comment", "positive"),
("This is a negative comment", "negative"),
("I'm not sure how I feel about this", "neutral"),
("Great product!", "positive"),
("Terrible customer service", "negative"),
("I would give this zero stars if I could", "negative"),
("Average at best", "neutral"),
("I love this company", "positive"),
("I hate this product", "negative"),
("Could be better", "neutral")]
# 将文本转换为向量
vectorizer = CountVectorizer()
text = [d[0] for d in data]
X = vectorizer.fit_transform(text)
# 训练贝叶斯分类器
y = [d[1] for d in data]
clf = MultinomialNB()
clf.fit(X, y)
# 对新评论进行分类
new_comment = "This is a great product!"
X_new = vectorizer.transform([new_comment])
y_pred = clf.predict(X_new)
print("Predicted sentiment:", y_pred[0])
```
以上就是一个基于Python语言的聚类 k-评论算法实例,使用了决策树和贝叶斯分类器进行分类。
相关推荐
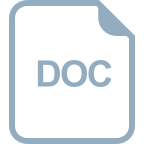
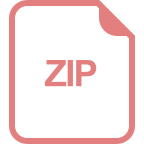
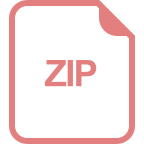
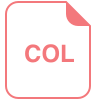
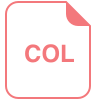
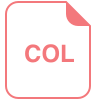
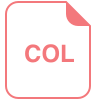
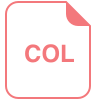









