vue2父组件怎么通过props动态获取子组件最新的值
时间: 2023-08-05 19:04:45 浏览: 85
在 Vue2 中,父组件可以通过监听子组件的事件或使用 `.sync` 修饰符来获取子组件的最新值。
1. 监听子组件的事件
子组件在需要更新父组件的值时,可以通过 `$emit` 方法触发一个自定义事件,并将需要传递的数据作为参数传递给父组件。父组件可以在模板中使用 `v-on` 或 `@` 来监听子组件的事件,并在事件处理函数中更新自己的值。
子组件:
```
<template>
<div>
<input type="text" v-model="value" @input="updateValue">
</div>
</template>
<script>
export default {
props: ['value'],
methods: {
updateValue(event) {
this.$emit('update:value', event.target.value)
}
}
}
</script>
```
父组件:
```
<template>
<div>
<child-component :value="parentValue" @update:value="updateParentValue"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue'
export default {
components: { ChildComponent },
data() {
return {
parentValue: ''
}
},
methods: {
updateParentValue(value) {
this.parentValue = value
}
}
}
</script>
```
2. 使用 .sync 修饰符
`.sync` 修饰符是 Vue2 中用于简化事件监听的一种方式,它可以让子组件更新父组件的值,并且可以通过 `v-bind` 的缩写语法来向子组件传递一个同名的 prop。在子组件中,可以通过 `$emit` 方法触发一个带有 `update:` 前缀的事件来更新父组件的值。
子组件:
```
<template>
<div>
<input type="text" :value="value" @input="$emit('update:value', $event.target.value)">
</div>
</template>
<script>
export default {
props: ['value']
}
</script>
```
父组件:
```
<template>
<div>
<child-component :value.sync="parentValue"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue'
export default {
components: { ChildComponent },
data() {
return {
parentValue: ''
}
}
}
</script>
```
无论使用哪种方式,父组件都可以获取到子组件最新的值。
阅读全文
相关推荐
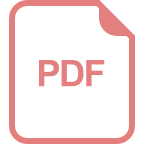
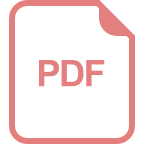














