jpeg图像锐化python代码
时间: 2023-10-13 09:20:22 浏览: 75
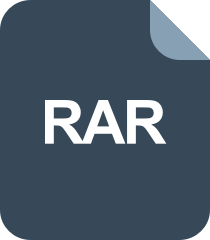
图片锐化程序

当然可以回答。以下是一段Python代码,可以对jpeg图像进行锐化:
```python
from PIL import Image
import numpy as np
def sharp_image(image_path):
img = Image.open(image_path)
img_arr = np.array(img)
kernel = np.array([[-1,-1,-1],[-1,9,-1],[-1,-1,-1]])
img_sharp = np.zeros_like(img_arr)
for i in range(3):
img_sharp[:,:,i] = np.clip(np.convolve(img_arr[:,:,i].ravel(), kernel.ravel(), mode="same").reshape(img_arr.shape[:-1]), 0, 255)
img_sharp = Image.fromarray(np.uint8(img_sharp))
img_sharp.show()
```
其中,我们使用了PIL库来打开和保存图像,并使用numpy库来处理图像数组。具体来说,我们使用了卷积运算来对图像进行锐化,使用的卷积核为:
```
[[-1,-1,-1],
[-1, 9,-1],
[-1,-1,-1]]
```
你可以将上述代码保存为一个.py文件,并在命令行或者编辑器中运行,然后传入jpeg图像的路径来测试我们的代码。
阅读全文
相关推荐
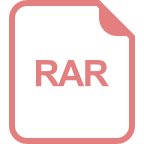
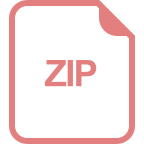
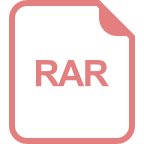
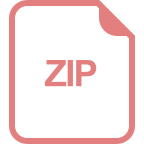
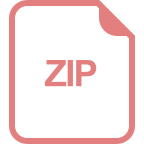
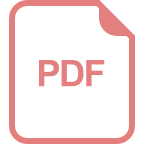
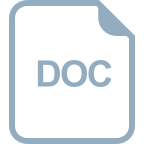
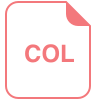
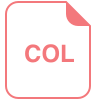
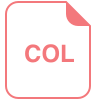
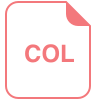
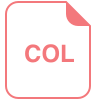
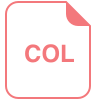
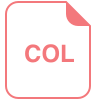
