<ItemsControl x:Class="HDSProject.Control.CustomFont" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:local="clr-namespace:HDSProject.Control" mc:Ignorable="d" d:DesignHeight="450" d:DesignWidth="800" x:Name="itemCtrl" ItemsSource="{Binding Path=Title2, RelativeSource={RelativeSource Mode=Self}}" FontSize="20" Foreground="Teal" > <ItemsControl.ItemContainerStyle> <Style TargetType="ContentPresenter"> <Setter Property="VerticalAlignment" Value="Center" /> </Style> </ItemsControl.ItemContainerStyle> <ItemsControl.ItemsPanel> <ItemsPanelTemplate> <StackPanel Orientation="Horizontal" HorizontalAlignment="Center"/> </ItemsPanelTemplate> </ItemsControl.ItemsPanel> <ItemsControl.ItemTemplate> <DataTemplate> <StackPanel> <Border Margin="{Binding Path=CharacterSpacing, RelativeSource={RelativeSource Mode=FindAncestor, AncestorType=local:CustomFont}}"> <TextBlock Text="{Binding}" /> </Border> <Rectangle Height="1" Fill="{Binding Foreground, ElementName=itemCtrl}" Margin="0,0,0,5"> <Rectangle.Style> <Style TargetType="Rectangle"> <Setter Property="Visibility" Value="Collapsed" /> <Style.Triggers> <DataTrigger Binding="{Binding ShowUnderline, RelativeSource={RelativeSource Mode=FindAncestor, AncestorType=local:CustomFont}}" Value="True"> <Setter Property="Visibility" Value="Visible" /> </DataTrigger> </Style.Triggers> </Style> </Rectangle.Style> </Rectangle> </StackPanel> </DataTemplate> </ItemsControl.ItemTemplate> </ItemsControl>添加Thumb控件来实现拉四条边改变控件大小
时间: 2024-02-14 09:10:25 浏览: 168
你的问题是如何添加Thumb控件来实现拉四条边改变控件大小。在WPF中,Thumb控件是用于拖动和调整元素大小的控件。要实现拖动和调整元素大小的功能,需要将Thumb控件添加到元素的四个边缘,并将Thumb的DragDelta事件处理程序与元素的大小和位置属性关联。
以下是添加Thumb控件的基本步骤:
1. 在元素的模板中添加四个Thumb控件,分别放置在元素的上、下、左、右四个边缘。
2. 将Thumb控件的DragDelta事件处理程序与元素的大小和位置属性关联,以便在拖动Thumb时更新元素的大小和位置。
3. 在Thumb控件的模板中设置Thumb的样式和大小。
以下是一个示例代码,演示如何添加Thumb控件来实现拖动和调整元素大小的功能:
```
<ControlTemplate TargetType="{x:Type local:CustomControl}">
<Grid>
<!-- 添加四个Thumb控件,分别放置在元素的上、下、左、右四个边缘 -->
<Thumb x:Name="TopThumb" DragDelta="TopThumb_DragDelta" Width="10" Height="10" HorizontalAlignment="Left" VerticalAlignment="Top" />
<Thumb x:Name="BottomThumb" DragDelta="BottomThumb_DragDelta" Width="10" Height="10" HorizontalAlignment="Left" VerticalAlignment="Bottom" />
<Thumb x:Name="LeftThumb" DragDelta="LeftThumb_DragDelta" Width="10" Height="10" HorizontalAlignment="Left" VerticalAlignment="Top" />
<Thumb x:Name="RightThumb" DragDelta="RightThumb_DragDelta" Width="10" Height="10" HorizontalAlignment="Right" VerticalAlignment="Top" />
<!-- 元素的内容 -->
<ContentPresenter />
</Grid>
</ControlTemplate>
```
在每个Thumb控件的DragDelta事件处理程序中,更新元素的大小和位置属性,以便在拖动Thumb时调整元素的大小和位置。
```
private void TopThumb_DragDelta(object sender, DragDeltaEventArgs e)
{
double oldHeight = Height;
double newHeight = oldHeight - e.VerticalChange;
double top = Top + e.VerticalChange;
if (newHeight > 0)
{
Height = newHeight;
Top = top;
}
}
private void BottomThumb_DragDelta(object sender, DragDeltaEventArgs e)
{
double newHeight = Height + e.VerticalChange;
if (newHeight > 0)
{
Height = newHeight;
}
}
private void LeftThumb_DragDelta(object sender, DragDeltaEventArgs e)
{
double oldWidth = Width;
double newWidth = oldWidth - e.HorizontalChange;
double left = Left + e.HorizontalChange;
if (newWidth > 0)
{
Width = newWidth;
Left = left;
}
}
private void RightThumb_DragDelta(object sender, DragDeltaEventArgs e)
{
double newWidth = Width + e.HorizontalChange;
if (newWidth > 0)
{
Width = newWidth;
}
}
```
注意,这只是一个简单的示例代码,实际情况可能更复杂。你需要根据实际需求和情况来调整代码。
阅读全文
相关推荐
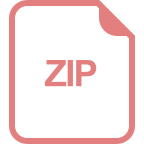
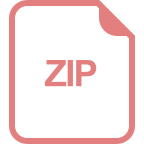
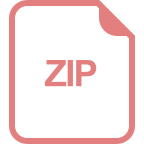





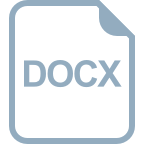
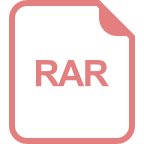
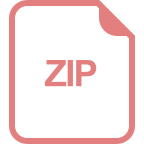
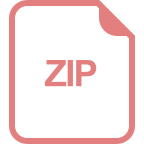
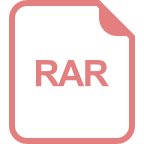