vc6.0rsa的数字签名C++
时间: 2023-07-11 16:20:47 浏览: 162
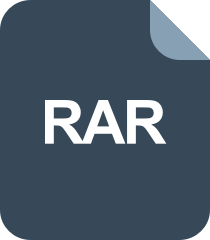
基于rsa数字签名c++实现

实现数字签名的方式有很多种,下面是使用 VC6.0 实现 RSA 数字签名的示例代码:
```cpp
#include <windows.h>
#include <wincrypt.h>
#include <stdio.h>
#include <string.h>
#pragma comment(lib, "Crypt32.lib")
DWORD MyHandleError(char *s);
int main()
{
HCRYPTPROV hProv = NULL;
HCRYPTHASH hHash = NULL;
HCRYPTKEY hKey = NULL;
DWORD dwSigLen = 0;
DWORD dwHashLen = 0;
BYTE pbHash[1024] = { 0 };
BYTE pbSignature[1024] = { 0 };
CHAR szContainerName[] = "MyContainer";
CHAR szProviderName[] = "Microsoft Enhanced Cryptographic Provider v1.0";
CHAR szData[] = "This is a test data for signing";
// acquire a handle to the default key container
if (!CryptAcquireContext(&hProv, szContainerName, szProviderName, PROV_RSA_FULL, 0))
{
if (GetLastError() == NTE_BAD_KEYSET)
{
// create a new key container
if (!CryptAcquireContext(&hProv, szContainerName, szProviderName, PROV_RSA_FULL, CRYPT_NEWKEYSET))
MyHandleError("Error in acquiring context");
}
else
{
MyHandleError("Error in acquiring context");
}
}
// create a hash object
if (!CryptCreateHash(hProv, CALG_SHA1, 0, 0, &hHash))
MyHandleError("Error in creating hash object");
// hash the data
if (!CryptHashData(hHash, (BYTE*)szData, strlen(szData), 0))
MyHandleError("Error in hashing data");
// determine the size of the signature
if (!CryptSignHash(hHash, AT_SIGNATURE, NULL, 0, NULL, &dwSigLen))
MyHandleError("Error in determining signature length");
// allocate memory for the signature
if (!CryptSignHash(hHash, AT_SIGNATURE, NULL, 0, pbSignature, &dwSigLen))
MyHandleError("Error in signing hash");
// verify the signature
if (!CryptGetUserKey(hProv, AT_SIGNATURE, &hKey))
MyHandleError("Error in getting user key");
if (!CryptVerifySignature(hHash, pbSignature, dwSigLen, hKey, NULL, 0))
MyHandleError("Error in verifying signature");
printf("Signature verified successfully\n");
// release resources
if (hKey)
CryptDestroyKey(hKey);
if (hHash)
CryptDestroyHash(hHash);
if (hProv)
CryptReleaseContext(hProv, 0);
return 0;
}
DWORD MyHandleError(char *s)
{
fprintf(stderr, "%s\n", s);
exit(EXIT_FAILURE);
}
```
这段代码会创建一个 RSA 密钥对,使用 SHA1 哈希算法对数据进行哈希,然后对哈希值进行数字签名,并验证签名的正确性。在实际使用中,可以根据需要修改哈希算法和签名算法,以及相关的参数。
阅读全文
相关推荐
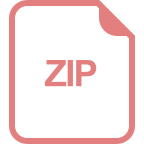
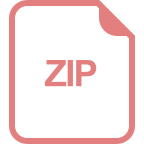

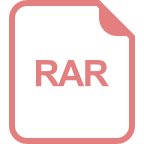
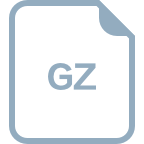
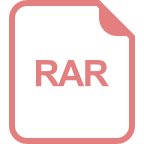
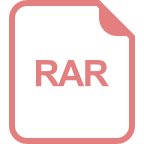
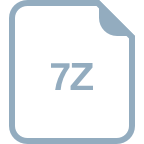
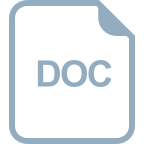
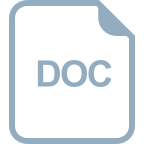
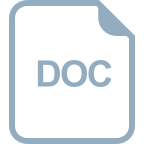
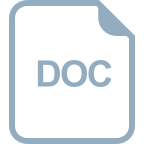
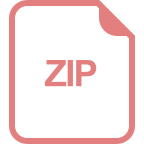
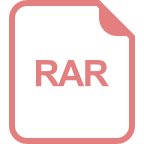
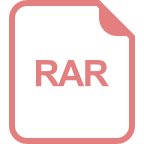
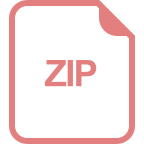
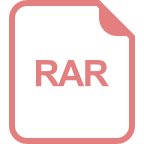
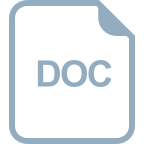