灰狼优化算法求解车间调度问题 代码python
时间: 2023-09-07 11:17:15 浏览: 135
以下是使用灰狼优化算法求解车间调度问题的 Python 代码:
```python
import numpy as np
# 车间调度问题的目标函数
def makespan(schedule, n_jobs, n_machines, processing_times):
start_times = np.zeros(n_jobs)
end_times = np.zeros(n_jobs)
machine_times = np.zeros(n_machines)
for i in schedule:
machine = np.argmin(machine_times)
start_times[i] = machine_times[machine]
machine_times[machine] += processing_times[i][machine]
end_times[i] = machine_times[machine]
return max(end_times)
# 灰狼优化算法
def grey_wolf_optimizer(obj_func, lb, ub, dim, n_pop, n_iter):
alpha = np.zeros(dim)
beta = np.zeros(dim)
delta = np.zeros(dim)
best_wolf = np.zeros(dim)
best_fitness = float('inf')
wolves = np.random.uniform(lb, ub, (n_pop, dim))
for i in range(n_iter):
for j in range(n_pop):
fitness = obj_func(wolves[j])
if fitness < best_fitness:
best_fitness = fitness
best_wolf = wolves[j]
a = 2 - 2 * (i / n_iter) # alpha 值逐渐减小
r1 = np.random.uniform()
r2 = np.random.uniform()
A = 2 * a * r1 - a
C = 2 * r2
D = np.abs(C * best_wolf - wolves[j])
X1 = best_wolf - A * D
r1 = np.random.uniform()
r2 = np.random.uniform()
A = 2 * a * r1 - a
C = 2 * r2
D = np.abs(C * alpha - wolves[j])
X2 = alpha - A * D
r1 = np.random.uniform()
r2 = np.random.uniform()
A = 2 * a * r1 - a
C = 2 * r2
D = np.abs(C * beta - wolves[j])
X3 = beta - A * D
wolves[j] = (X1 + X2 + X3) / 3
# 更新 alpha, beta, delta
if fitness < obj_func(alpha):
alpha = wolves[j]
if obj_func(alpha) < fitness < obj_func(beta):
beta = wolves[j]
if obj_func(alpha) < obj_func(delta) < obj_func(beta):
delta = wolves[j]
return best_wolf
# 测试
n_jobs = 5
n_machines = 3
processing_times = np.array([[4, 7, 3], [2, 4, 5], [9, 3, 6], [8, 2, 1], [5, 5, 5]])
def obj_func(x):
schedule = np.argsort(x)
return makespan(schedule, n_jobs, n_machines, processing_times)
lb = np.zeros(n_jobs)
ub = n_machines * np.ones(n_jobs)
dim = n_jobs
n_pop = 30
n_iter = 100
best_wolf = grey_wolf_optimizer(obj_func, lb, ub, dim, n_pop, n_iter)
print(best_wolf)
```
代码中使用了 NumPy 库来方便地进行矩阵运算。在测试部分,我们定义了一个 5 个工件和 3 个机器的车间调度问题,并通过灰狼优化算法求解最小化的完工时间。最终输出的是最优解对应的工件调度顺序。
阅读全文
相关推荐
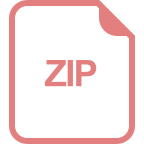
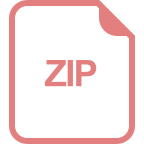
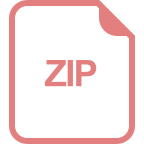
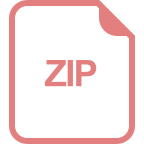
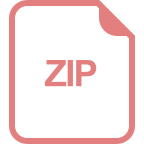
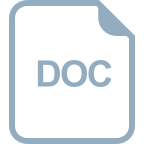
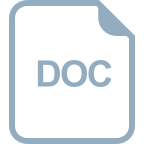
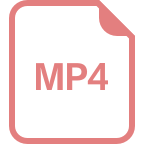
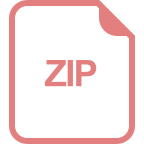
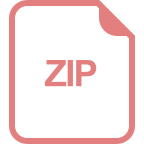
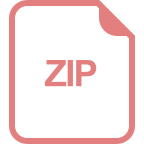
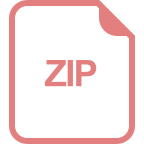
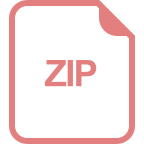
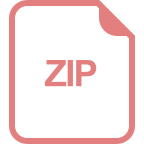
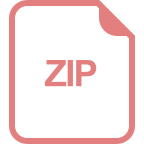
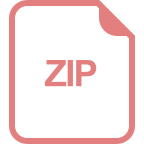
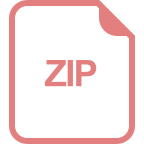