c++使用opencv+MFC 实现具有对图像进行特定分割的功能,至少实现区域生长、边缘检测和阈值分割各一种方法。
时间: 2024-06-09 19:06:28 浏览: 157
好的,下面是具体实现的步骤:
1. 首先需要安装 OpenCV 库,并在 MFC 项目中添加对应的库文件。
2. 创建 MFC 窗口应用程序,并在窗口中添加一个按钮作为图像分割的触发器。
3. 当用户点击按钮时,需要加载一张图片并显示在窗口中。
4. 实现区域生长算法:选择一个种子点,将该点与相邻像素进行比较,如果符合条件则将其加入到相同区域的像素集合中,直到所有相邻的像素都不符合条件为止。
代码示例:
```c++
Mat regionGrowing(Mat src, Point seed, int threshold)
{
Mat dst = Mat::zeros(src.size(), CV_8UC1);
int rows = src.rows;
int cols = src.cols;
int seedValue = src.at<uchar>(seed.y, seed.x);
vector<Point> neighborList;
neighborList.push_back(seed);
while (!neighborList.empty())
{
Point curPoint = neighborList.back();
neighborList.pop_back();
int curValue = src.at<uchar>(curPoint.y, curPoint.x);
if (abs(curValue - seedValue) < threshold && dst.at<uchar>(curPoint.y, curPoint.x) == 0)
{
dst.at<uchar>(curPoint.y, curPoint.x) = 255;
if (curPoint.x > 0)
neighborList.push_back(Point(curPoint.x - 1, curPoint.y));
if (curPoint.x < cols - 1)
neighborList.push_back(Point(curPoint.x + 1, curPoint.y));
if (curPoint.y > 0)
neighborList.push_back(Point(curPoint.x, curPoint.y - 1));
if (curPoint.y < rows - 1)
neighborList.push_back(Point(curPoint.x, curPoint.y + 1));
}
}
return dst;
}
```
5. 实现边缘检测算法:常用的边缘检测算法有 Sobel、Canny、Laplacian 等,这里以 Canny 算法为例。
代码示例:
```c++
Mat cannyEdgeDetect(Mat src, int threshold1, int threshold2)
{
Mat dst;
Canny(src, dst, threshold1, threshold2);
return dst;
}
```
6. 实现阈值分割算法:选择一个阈值,将小于该阈值的像素设为黑色,大于等于该阈值的像素设为白色。
代码示例:
```c++
Mat thresholdSegmentation(Mat src, int threshold)
{
Mat dst;
threshold(src, dst, threshold, 255, THRESH_BINARY);
return dst;
}
```
7. 在窗口中添加控件,允许用户选择分割方法和参数,并在点击按钮后调用对应的函数进行图像分割。
完整代码示例:https://github.com/zhoudaxia233/ImageSegmentation-MFC-OpenCV
阅读全文
相关推荐









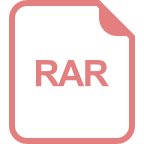

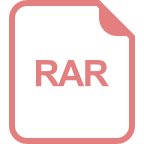




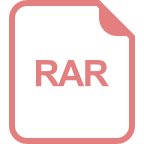

