表单的方法请求添加header
时间: 2023-10-08 08:11:39 浏览: 148
可以使用 `fetch` 方法进行表单的方法请求,并通过传递一个包含 header 信息的对象来添加 header。例如,以下代码可以使用 POST 方法向服务器发送一个包含 header 的表单请求:
```
fetch('/submit-form', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer my-token'
},
body: JSON.stringify(formData)
})
```
其中,`headers` 对象包含了两个键值对,分别是 `Content-Type` 和 `Authorization`。`Content-Type` 表示请求体的类型,这里使用的是 JSON 格式;`Authorization` 表示身份认证信息,这里使用的是 Bearer token。你可以根据实际情况修改这些值。
相关问题
form表单设置post请求头
在 HTML 表单中,设置 POST 请求头通常不需要直接在表单元素上进行操作,因为浏览器会默认发送 GET 或 POST 请求。但是,如果你想在发送表单数据到后端服务时添加自定义的请求头信息,可以借助 JavaScript 或者后端编程语言来实现。
**JavaScript 示例**(前端):
```javascript
var xhr = new XMLHttpRequest();
xhr.open('POST', 'your-url-here', true);
xhr.setRequestHeader('Content-Type', 'application/json'); // 设置请求头 Content-Type 为 json
xhr.setRequestHeader('Authorization', 'Bearer your-token'); // 添加其他自定义头信息
// 发送表单数据
xhr.send(JSON.stringify({
key: value,
... // 表单数据
}));
```
在这个例子中,我们使用了 `XMLHttpRequest` 对象手动构造了一个 POST 请求,并设置了 `setRequestHeader` 方法来添加请求头。
**后端示例**(Node.js 使用 Express为例):
```javascript
const express = require('express');
const app = express();
app.use(express.json()); // 配置中间件来解析 JSON 格式请求体
app.post('/your-endpoint', function(req, res) {
const token = req.headers.authorization; // 获取 Authorization 请求头
console.log('Received POST request with custom header:', token);
// 处理请求...
});
```
在后端,你可以通过 `req.headers` 对象访问到来的请求头。
需要注意的是,某些敏感信息(如 Token)可能不适合放在请求头里,而是应该作为 URL 参数或者通过某种加密方式放置在请求体中。同时,不是所有后端服务器都会接受来自客户端的所有请求头,所以最好查阅服务器文档了解支持哪些头部字段。
请求体怎么写?请给出header和body的示例
在C#中,创建HTTP请求体(RequestBody)取决于你想要发送的数据类型。例如,最常见的数据类型有文本(如JSON)、XML、表单数据等。
**文本数据(JSON)为例:**
如果你想发送JSON格式的数据,可以使用`StringContent`类配合`JsonConvert.SerializeObject`将对象转换成字符串,然后设置到请求体:
```csharp
string jsonPayload = JsonConvert.SerializeObject(yourObject); // 将对象序列化为JSON字符串
using (var content = new StringContent(jsonPayload, Encoding.UTF8, "application/json"))
{
// 发送POST请求
var response = await client.PostAsync("https://api.example.com/resource", content);
}
```
这里`yourObject`是你想要发送的.NET对象,如`Dictionary<string, object>`或自定义类。
**HTTP Header 示例:**
HTTP头部可以包含多种信息,如身份认证、内容编码等。设置头部通常是这样的:
```csharp
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Put, "https://example.com/data");
request.Headers.Authorization = new AuthenticationHeaderValue("Bearer", "your_access_token"); // 设置访问令牌
request.Headers.ContentType = MediaTypeHeaderValue.Parse("application/json"); // 告诉服务器你正在发送的是JSON
```
这里的`AuthenticationHeaderValue`是添加Authorization字段,`MediaTypeHeaderValue`表示数据类型。
阅读全文
相关推荐
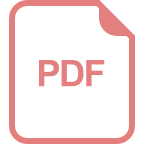
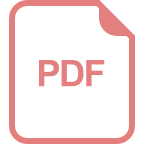
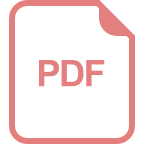
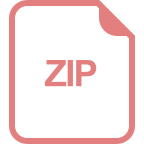
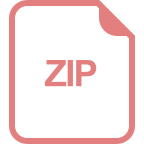
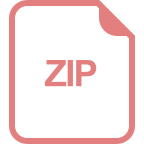
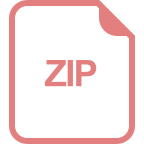
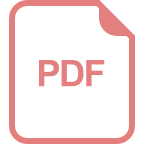
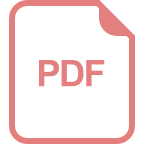
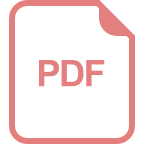
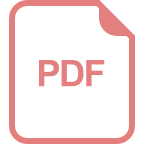
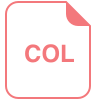
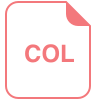
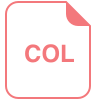


