用c#设计一个计算bmi值的程序,并给出wpf框架
时间: 2024-02-15 19:01:48 浏览: 144
好的,以下是一个使用WPF框架设计的计算BMI值的程序:
首先,我们需要在XAML中设计用户界面。在这里,我们将使用两个文本框和一个按钮来允许用户输入体重和身高,然后计算BMI值并显示结果。
```xaml
<Window x:Class="BMICalculator.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="BMI Calculator" Height="250" Width="300">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<Label Grid.Row="0" Content="Weight (kg):"/>
<TextBox Grid.Row="0" Name="weightTextBox" Margin="5,0,0,0" />
<Label Grid.Row="1" Content="Height (m):"/>
<TextBox Grid.Row="1" Name="heightTextBox" Margin="5,0,0,0" />
<Button Grid.Row="2" Content="Calculate BMI" Name="calculateButton" Margin="5,10,5,0" Click="CalculateButton_Click"/>
<Label Grid.Row="3" Name="bmiResultLabel" Margin="5,10,5,5"/>
</Grid>
</Window>
```
然后,我们需要编写C#代码来计算BMI值并将其显示在标签中。在这个例子中,我们将在单击“计算BMI”按钮时执行计算,并将结果显示在一个标签中。
```csharp
using System;
using System.Windows;
namespace BMICalculator
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void CalculateButton_Click(object sender, RoutedEventArgs e)
{
try
{
double weight = double.Parse(weightTextBox.Text);
double height = double.Parse(heightTextBox.Text);
double bmi = weight / (height * height);
bmiResultLabel.Content = "Your BMI is: " + bmi.ToString("F2");
}
catch (FormatException)
{
bmiResultLabel.Content = "Please enter valid numbers for weight and height.";
}
}
}
}
```
这个程序很简单:当用户单击“计算BMI”按钮时,它将读取文本框中的体重和身高值,并使用这些值计算BMI。然后,它将BMI值显示在一个标签中。如果用户输入的不是数字,它将显示一个错误提示。
希望这可以帮助您开始编写BMI计算器。
阅读全文
相关推荐




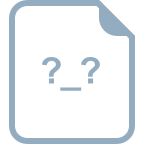




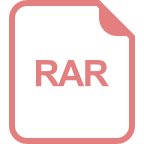





