STM32步进电机加减速运动的程序该怎么写
时间: 2023-10-03 21:08:32 浏览: 94
下面是一个基本的STM32步进电机加减速运动的程序框架:
```c
#include "stm32f4xx.h"
#define MOTOR_STEP_PIN GPIO_Pin_0
#define MOTOR_DIR_PIN GPIO_Pin_1
#define ACCELERATION 1000 // 加速度
#define MAX_SPEED 5000 // 最大速度
#define MIN_SPEED 1000 // 最小速度
#define SPEED_INTERVAL 100 // 速度间隔
uint32_t current_speed = 0; // 当前速度
uint32_t target_speed = 0; // 目标速度
uint32_t acceleration = 0; // 当前加速度
uint8_t direction = 0; // 运动方向,0为正向,1为反向
void motor_init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = MOTOR_STEP_PIN | MOTOR_DIR_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
void motor_step(void)
{
GPIO_SetBits(GPIOA, MOTOR_STEP_PIN);
GPIO_ResetBits(GPIOA, MOTOR_STEP_PIN);
}
void motor_move(uint8_t dir)
{
if (dir == 0) {
GPIO_ResetBits(GPIOA, MOTOR_DIR_PIN);
direction = 0;
} else {
GPIO_SetBits(GPIOA, MOTOR_DIR_PIN);
direction = 1;
}
}
void motor_stop(void)
{
current_speed = 0;
target_speed = 0;
acceleration = 0;
direction = 0;
}
void motor_set_speed(uint32_t speed)
{
if (speed > MAX_SPEED)
speed = MAX_SPEED;
if (speed < MIN_SPEED)
speed = MIN_SPEED;
target_speed = speed;
acceleration = ACCELERATION;
}
void motor_move_to(uint32_t pos)
{
// 计算速度
motor_set_speed((pos > SPEED_INTERVAL) ? pos / SPEED_INTERVAL : MIN_SPEED);
}
void motor_update(void)
{
if (current_speed < target_speed) {
current_speed += acceleration;
if (current_speed > target_speed)
current_speed = target_speed;
}
if (current_speed > target_speed) {
current_speed -= acceleration;
if (current_speed < target_speed)
current_speed = target_speed;
}
if (current_speed == 0)
return;
uint32_t delay_us = 1000000 / current_speed;
for (uint32_t i = 0; i < 200; i++) {
motor_step();
delay_us(delay_us);
}
}
int main(void)
{
motor_init();
while (1) {
motor_update();
}
return 0;
}
```
程序中使用了一个简单的加减速算法来控制速度,当速度达到目标速度时,就开始运动。每次运动时,先更新速度,然后计算出每步的延迟时间,最后根据延迟时间循环运动电机。这个程序只是一个简单的框架,具体的实现需要根据具体的需求进行调整。
阅读全文
相关推荐
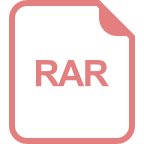
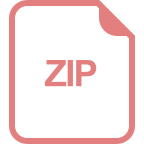
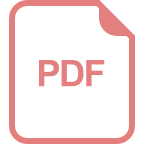
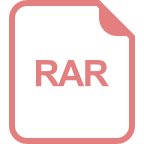
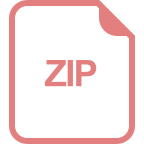
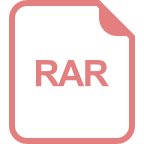
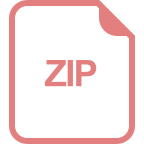
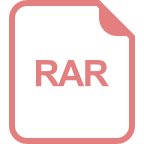




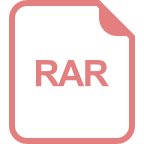
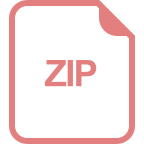