hadoop集群环境下。使用Java代码进行数据求和的程序
时间: 2024-02-19 07:02:00 浏览: 23
如果你想在Hadoop集群环境下使用Java代码进行数据求和的程序,可以按照以下步骤进行:
1. 编写Mapper类
Mapper类的作用是读取输入数据并将其转换为键值对。在本例中,我们可以将每个数字映射为一个键值对,其中键为固定的字符串(比如"sum"),值为该数字。
```java
import org.apache.hadoop.io.*;
import org.apache.hadoop.mapreduce.*;
public class SumMapper extends Mapper<LongWritable, Text, Text, LongWritable> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
LongWritable number = new LongWritable(Long.parseLong(value.toString()));
context.write(new Text("sum"), number);
}
}
```
2. 编写Reducer类
Reducer类的作用是对Mapper类输出的键值对进行归约操作。在本例中,我们可以对相同键的数字进行求和操作。
```java
import org.apache.hadoop.io.*;
import org.apache.hadoop.mapreduce.*;
public class SumReducer extends Reducer<Text, LongWritable, Text, LongWritable> {
public void reduce(Text key, Iterable<LongWritable> values, Context context) throws IOException, InterruptedException {
long sum = 0;
for (LongWritable value : values) {
sum += value.get();
}
context.write(key, new LongWritable(sum));
}
}
```
3. 编写Driver类
Driver类的作用是将Mapper类和Reducer类组合在一起,并指定输入数据和输出路径等参数。
```java
import org.apache.hadoop.conf.*;
import org.apache.hadoop.fs.*;
import org.apache.hadoop.io.*;
import org.apache.hadoop.mapreduce.*;
public class SumDriver {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "sum");
job.setJarByClass(SumDriver.class);
job.setMapperClass(SumMapper.class);
job.setReducerClass(SumReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(LongWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
4. 执行程序
在Hadoop集群环境下,可以使用以下命令执行程序:
```
hadoop jar sum.jar SumDriver input output
```
其中,`sum.jar`为打包好的程序包,`input`为输入数据的路径,`output`为输出结果的路径。
相关推荐
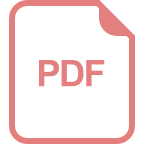
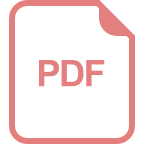














