我需要一个监控线程的线程,以重启死了的线程
时间: 2023-11-28 19:08:12 浏览: 63
可以使用 Python 的 threading 模块实现一个监控线程的线程。具体步骤如下:
1. 定义一个线程类 MyThread,继承自 threading.Thread;
2. 在 MyThread 中定义一个成员变量 running,表示线程是否正在运行;
3. 在 MyThread 中重写 run() 方法,其中使用 while 循环不断检测被监控线程的状态,如果发现被监控线程已经退出,则重新启动被监控线程;
4. 在主线程中创建 MyThread 对象,并启动该线程和需要被监控的线程。
以下是示例代码:
```python
import threading
import time
class MyThread(threading.Thread):
def __init__(self, thread_func):
super().__init__()
self.thread_func = thread_func
self.running = True
def run(self):
while self.running:
if not self.thread_func.is_alive():
print("Thread is dead. Restarting...")
self.thread_func.start()
time.sleep(1)
def stop(self):
self.running = False
def monitored_thread():
while True:
print("Hello from monitored thread!")
time.sleep(5)
if __name__ == "__main__":
t = threading.Thread(target=monitored_thread)
t.start()
monitor_thread = MyThread(t)
monitor_thread.start()
time.sleep(20)
monitor_thread.stop()
t.join()
```
在上面的示例中,我们创建了一个被监控的线程 monitored_thread,这个线程每隔 5 秒输出一句话。同时,我们创建了一个 MyThread 实例 monitor_thread,监控 monitored_thread 的状态。主线程先启动 monitored_thread,然后启动 monitor_thread。在主线程中等待 20 秒后,停止 monitor_thread 和 monitored_thread。
阅读全文
相关推荐
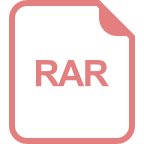
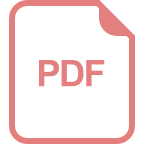
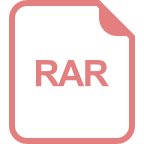
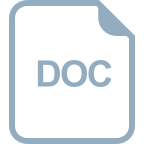

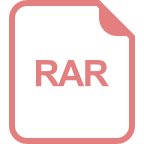
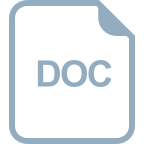
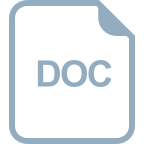
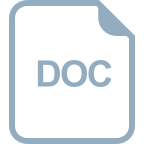
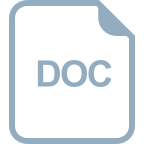
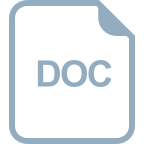
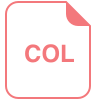
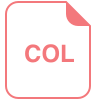





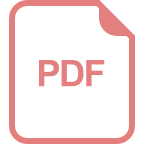