通过c++使用链表设计一个学生成绩管理系统,通过加权平均的方法计算平均学分绩点,同时包括学生信息的添加删除以及各课程的成绩排序和平均学分绩点排序
时间: 2024-02-13 12:06:13 浏览: 55
好的,我会尽力回答你的问题。
首先,我们需要定义一个学生类,包括学生基本信息和成绩信息。然后,我们可以使用链表来存储所有学生的信息,包括每个学生的成绩信息。
以下是一个可能的学生类的示例:
```cpp
class Student {
public:
string name;
int id;
float grades[10];
float credits[10];
float gpa;
Student* next;
// constructor
Student(string name, int id, float grades[], float credits[]) {
this->name = name;
this->id = id;
for (int i = 0; i < 10; i++) {
this->grades[i] = grades[i];
this->credits[i] = credits[i];
}
this->gpa = calculateGPA();
this->next = nullptr;
}
// calculate GPA
float calculateGPA() {
float totalGPA = 0.0;
float totalCredits = 0.0;
for (int i = 0; i < 10; i++) {
totalGPA += grades[i] * credits[i];
totalCredits += credits[i];
}
return totalGPA / totalCredits;
}
};
```
接下来,我们可以使用一个链表来存储所有的学生信息。这里我们使用单向链表,并且添加了一些基本的操作,如添加、删除和遍历链表等。
```cpp
class StudentList {
public:
Student* head;
// constructor
StudentList() {
this->head = nullptr;
}
// add a student to list
void addStudent(string name, int id, float grades[], float credits[]) {
Student* newStudent = new Student(name, id, grades, credits);
if (head == nullptr) {
head = newStudent;
} else {
Student* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newStudent;
}
}
// remove a student from list by id
void removeStudent(int id) {
if (head == nullptr) {
return;
}
if (head->id == id) {
Student* temp = head;
head = head->next;
delete temp;
return;
}
Student* current = head;
while (current->next != nullptr) {
if (current->next->id == id) {
Student* temp = current->next;
current->next = temp->next;
delete temp;
return;
}
current = current->next;
}
}
// sort students by a specific course
void sortByCourse(int course) {
if (head == nullptr) {
return;
}
bool swapped;
do {
swapped = false;
Student* current = head;
while (current->next != nullptr) {
if (current->grades[course] < current->next->grades[course]) {
swap(current, current->next);
swapped = true;
}
current = current->next;
}
} while (swapped);
}
// sort students by GPA
void sortByGPA() {
if (head == nullptr) {
return;
}
bool swapped;
do {
swapped = false;
Student* current = head;
while (current->next != nullptr) {
if (current->gpa < current->next->gpa) {
swap(current, current->next);
swapped = true;
}
current = current->next;
}
} while (swapped);
}
// swap two students
void swap(Student* a, Student* b) {
string tempName = a->name;
int tempId = a->id;
float tempGrades[10];
float tempCredits[10];
for (int i = 0; i < 10; i++) {
tempGrades[i] = a->grades[i];
tempCredits[i] = a->credits[i];
}
float tempGPA = a->gpa;
a->name = b->name;
a->id = b->id;
for (int i = 0; i < 10; i++) {
a->grades[i] = b->grades[i];
a->credits[i] = b->credits[i];
}
a->gpa = b->gpa;
b->name = tempName;
b->id = tempId;
for (int i = 0; i < 10; i++) {
b->grades[i] = tempGrades[i];
b->credits[i] = tempCredits[i];
}
b->gpa = tempGPA;
}
// calculate average GPA
float calculateAverageGPA() {
if (head == nullptr) {
return 0.0;
}
float totalGPA = 0.0;
int count = 0;
Student* current = head;
while (current != nullptr) {
totalGPA += current->gpa;
count++;
current = current->next;
}
return totalGPA / count;
}
};
```
现在,我们可以使用上面的类来创建一个学生成绩管理系统,并使用加权平均的方法计算平均学分绩点,同时包括学生信息的添加删除以及各课程的成绩排序和平均学分绩点排序。
```cpp
int main() {
StudentList list;
// add some students
float grades1[10] = { 90, 85, 95, 80, 88, 92, 90, 85, 95, 80 };
float credits1[10] = { 4, 3, 3, 3, 2, 2, 4, 3, 3, 3 };
list.addStudent("Tom", 1001, grades1, credits1);
float grades2[10] = { 85, 80, 90, 75, 83, 87, 85, 80, 90, 75 };
float credits2[10] = { 3, 3, 3, 3, 2, 2, 4, 3, 3, 3 };
list.addStudent("Jerry", 1002, grades2, credits2);
float grades3[10] = { 92, 87, 97, 82, 90, 94, 92, 87, 97, 82 };
float credits3[10] = { 4, 3, 3, 3, 2, 2, 4, 3, 3, 3 };
list.addStudent("Alice", 1003, grades3, credits3);
// remove a student
list.removeStudent(1002);
// sort students by a specific course
list.sortByCourse(0); // sort by the first course
// sort students by GPA
list.sortByGPA();
// calculate average GPA
float avgGPA = list.calculateAverageGPA();
return 0;
}
```
这样,我们就完成了一个使用链表设计的学生成绩管理系统。希望能对你有所帮助!
阅读全文
相关推荐
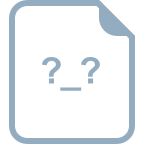
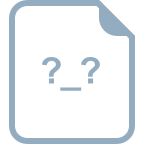
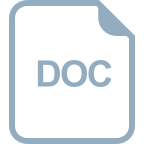
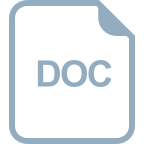
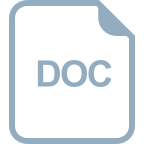
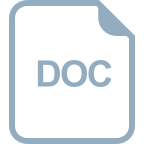

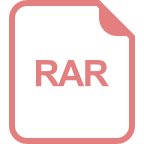
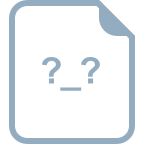
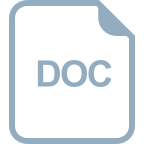
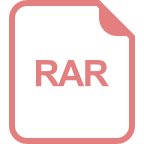
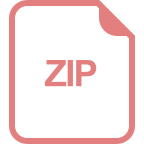
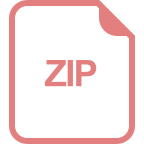
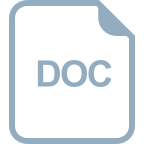