c++坦克大战代码实现
时间: 2023-08-03 12:39:17 浏览: 103
C++坦克大战的代码实现比较复杂,需要使用图形库来实现游戏界面的设计。下面是一个简单的C++坦克大战代码实现示例,仅供参考:
```c++
#include <graphics.h>
#include <conio.h>
#include <stdlib.h>
#include <time.h>
#define WIN_WIDTH 800
#define WIN_HEIGHT 600
#define BATTLE_WIDTH 640
#define BATTLE_HEIGHT 480
#define CELL_SIZE 16
#define TANK_SIZE 32
#define MAX_BULLETS 10
#define MAX_ENEMIES 5
struct point {
int x;
int y;
};
struct bullet {
point pos;
int direction;
};
struct tank {
point pos;
int direction;
int speed;
bullet bullets[MAX_BULLETS];
int bullet_count;
};
struct enemy {
point pos;
int direction;
int speed;
int alive;
};
void draw_map();
void draw_tank(tank t);
void draw_bullet(bullet b);
void draw_enemy(enemy e);
void move_tank(tank &t);
void move_bullet(bullet &b);
void move_enemy(enemy &e);
void fire_bullet(tank &t);
void fire_enemy(enemy &e);
int check_collision(point p, int w, int h);
int check_enemy_collision(tank &t);
int check_tank_collision(tank &t1, tank &t2);
int check_bullet_collision(tank &t, enemy &e);
int main() {
int gd = DETECT, gm;
initgraph(&gd, &gm, "");
setbkcolor(WHITE);
cleardevice();
setcolor(BLACK);
tank player;
player.pos.x = 0;
player.pos.y = 0;
player.direction = 0;
player.speed = 5;
player.bullet_count = 0;
enemy enemies[MAX_ENEMIES];
for (int i = 0; i < MAX_ENEMIES; i++) {
enemies[i].alive = 1;
enemies[i].direction = rand() % 4;
enemies[i].speed = 2;
enemies[i].pos.x = rand() % (BATTLE_WIDTH - TANK_SIZE);
enemies[i].pos.y = rand() % (BATTLE_HEIGHT - TANK_SIZE);
}
while (1) {
draw_map();
draw_tank(player);
for (int i = 0; i < player.bullet_count; i++) {
draw_bullet(player.bullets[i]);
move_bullet(player.bullets[i]);
}
for (int i = 0; i < MAX_ENEMIES; i++) {
if (enemies[i].alive) {
draw_enemy(enemies[i]);
move_enemy(enemies[i]);
if (check_bullet_collision(player, enemies[i])) {
enemies[i].alive = 0;
}
}
}
if (kbhit()) {
char ch = getch();
switch (ch) {
case 'w':
player.direction = 0;
move_tank(player);
break;
case 's':
player.direction = 2;
move_tank(player);
break;
case 'a':
player.direction = 3;
move_tank(player);
break;
case 'd':
player.direction = 1;
move_tank(player);
break;
case ' ':
fire_bullet(player);
break;
}
}
for (int i = 0; i < MAX_ENEMIES; i++) {
if (enemies[i].alive) {
fire_enemy(enemies[i]);
}
}
delay(30);
cleardevice();
}
return 0;
}
void draw_map() {
setcolor(BLACK);
rectangle(0, 0, BATTLE_WIDTH, BATTLE_HEIGHT);
rectangle(BATTLE_WIDTH, 0, WIN_WIDTH, WIN_HEIGHT);
outtextxy(BATTLE_WIDTH + 20, 20, "SCORE:");
outtextxy(BATTLE_WIDTH + 20, 50, "LIFE:");
}
void draw_tank(tank t) {
setfillstyle(SOLID_FILL, YELLOW);
switch (t.direction) {
case 0:
bar(t.pos.x, t.pos.y, t.pos.x + TANK_SIZE, t.pos.y + CELL_SIZE);
break;
case 1:
bar(t.pos.x + TANK_SIZE - CELL_SIZE, t.pos.y, t.pos.x + TANK_SIZE, t.pos.y + TANK_SIZE);
break;
case 2:
bar(t.pos.x, t.pos.y + TANK_SIZE - CELL_SIZE, t.pos.x + TANK_SIZE, t.pos.y + TANK_SIZE);
break;
case 3:
bar(t.pos.x, t.pos.y, t.pos.x + CELL_SIZE, t.pos.y + TANK_SIZE);
break;
}
}
void draw_bullet(bullet b) {
setfillstyle(SOLID_FILL, BLACK);
bar(b.pos.x, b.pos.y, b.pos.x + CELL_SIZE, b.pos.y + CELL_SIZE);
}
void draw_enemy(enemy e) {
setfillstyle(SOLID_FILL, RED);
switch (e.direction) {
case 0:
bar(e.pos.x, e.pos.y, e.pos.x + TANK_SIZE, e.pos.y + CELL_SIZE);
break;
case 1:
bar(e.pos.x + TANK_SIZE - CELL_SIZE, e.pos.y, e.pos.x + TANK_SIZE, e.pos.y + TANK_SIZE);
break;
case 2:
bar(e.pos.x, e.pos.y + TANK_SIZE - CELL_SIZE, e.pos.x + TANK_SIZE, e.pos.y + TANK_SIZE);
break;
case 3:
bar(e.pos.x, e.pos.y, e.pos.x + CELL_SIZE, e.pos.y + TANK_SIZE);
break;
}
}
void move_tank(tank &t) {
int old_x = t.pos.x;
int old_y = t.pos.y;
switch (t.direction) {
case 0:
t.pos.y -= t.speed;
break;
case 1:
t.pos.x += t.speed;
break;
case 2:
t.pos.y += t.speed;
break;
case 3:
t.pos.x -= t.speed;
break;
}
if (check_tank_collision(t, t)) {
t.pos.x = old_x;
t.pos.y = old_y;
}
}
void move_bullet(bullet &b) {
switch (b.direction) {
case 0:
b.pos.y -= 10;
break;
case 1:
b.pos.x += 10;
break;
case 2:
b.pos.y += 10;
break;
case 3:
b.pos.x -= 10;
break;
}
if (check_collision(b.pos, CELL_SIZE, CELL_SIZE)) {
b.pos.x = -10;
b.pos.y = -10;
}
}
void move_enemy(enemy &e) {
int old_x = e.pos.x;
int old_y = e.pos.y;
switch (e.direction) {
case 0:
e.pos.y -= e.speed;
break;
case 1:
e.pos.x += e.speed;
break;
case 2:
e.pos.y += e.speed;
break;
case 3:
e.pos.x -= e.speed;
break;
}
if (check_enemy_collision(e)) {
e.pos.x = old_x;
e.pos.y = old_y;
e.direction = rand() % 4;
}
}
void fire_bullet(tank &t) {
if (t.bullet_count == MAX_BULLETS) {
return;
}
bullet b;
b.pos.x = t.pos.x + TANK_SIZE / 2 - CELL_SIZE / 2;
b.pos.y = t.pos.y + TANK_SIZE / 2 - CELL_SIZE / 2;
b.direction = t.direction;
t.bullets[t.bullet_count++] = b;
}
void fire_enemy(enemy &e) {
if (rand() % 50 == 0) {
bullet b;
b.pos.x = e.pos.x + TANK_SIZE / 2 - CELL_SIZE / 2;
b.pos.y = e.pos.y + TANK_SIZE / 2 - CELL_SIZE / 2;
b.direction = e.direction;
}
}
int check_collision(point p, int w, int h) {
if (p.x < 0 || p.y < 0 || p.x + w > BATTLE_WIDTH || p.y + h > BATTLE_HEIGHT) {
return 1;
}
return 0;
}
int check_enemy_collision(tank &t) {
for (int i = 0; i < MAX_ENEMIES; i++) {
if (t.pos.x < enemies[i].pos.x + TANK_SIZE &&
t.pos.x + TANK_SIZE > enemies[i].pos.x &&
t.pos.y < enemies[i].pos.y + TANK_SIZE &&
t.pos.y + TANK_SIZE > enemies[i].pos.y) {
return 1;
}
}
return 0;
}
int check_tank_collision(tank &t1, tank &t2) {
if (t1.pos.x < t2.pos.x + TANK_SIZE &&
t1.pos.x + TANK_SIZE > t2.pos.x &&
t1.pos.y < t2.pos.y + TANK_SIZE &&
t1.pos.y + TANK_SIZE > t2.pos.y) {
return 1;
}
return 0;
}
int check_bullet_collision(tank &t, enemy &e) {
for (int i = 0; i < t.bullet_count; i++) {
if (t.bullets[i].pos.x < e.pos.x + TANK_SIZE &&
t.bullets[i].pos.x + CELL_SIZE > e.pos.x &&
t.bullets[i].pos.y < e.pos.y + TANK_SIZE &&
t.bullets[i].pos.y + CELL_SIZE > e.pos.y) {
t.bullets[i].pos.x = -10;
t.bullets[i].pos.y = -10;
t.bullet_count--;
return 1;
}
}
return 0;
}
```
这个代码实现了基本的游戏功能,包括坦克的移动、炮弹的发射、敌人的生成、敌人的移动和炮弹的碰撞检测等。但是代码实现还不够完善,需要根据实际情况进行调整和完善。
相关推荐
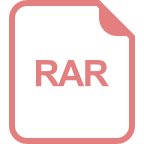
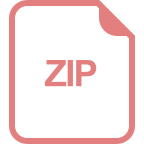















