Write a class named ProductSystem, which extends JFrame class. The appearance of the application looks like the following (as shown in Figure 1). 2. At the beginning, input name,price and number at three JTextField fields, then click “Add”button (event source), which binds to a method (event listener) that create a Product object (using name,price and number) and add it into ArrayList<Product>, at the same time display “Add Product xxx sucess!” in JTextArea on the GUI (as shown in Figure 2) 3. When click “Display” button, then read the records from ArrayList , and display the records in JTextArea on the GUI(as shown in Figure 3). 4. When click “Save to File” button, then write the records from ArrayList to file product.txt, at the same time display "file write success" in JTextArea on the GUI (as shown in Figure 4)
时间: 2024-03-08 13:46:08 浏览: 105
以下是一个实现您所需功能的 ProductSystem 类的示例代码:
```
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.*;
import javax.swing.*;
public class ProductSystem extends JFrame implements ActionListener {
private JLabel nameLabel, priceLabel, numberLabel;
private JTextField nameField, priceField, numberField;
private JButton addButton, displayButton, saveButton;
private JTextArea outputArea;
private ArrayList<Product> productList;
public ProductSystem() {
super("Product System");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(600, 400);
setLayout(new GridLayout(2, 1));
JPanel inputPanel = new JPanel(new GridLayout(3, 2));
nameLabel = new JLabel("Name:");
nameField = new JTextField();
priceLabel = new JLabel("Price:");
priceField = new JTextField();
numberLabel = new JLabel("Number:");
numberField = new JTextField();
addButton = new JButton("Add");
addButton.addActionListener(this);
inputPanel.add(nameLabel);
inputPanel.add(nameField);
inputPanel.add(priceLabel);
inputPanel.add(priceField);
inputPanel.add(numberLabel);
inputPanel.add(numberField);
inputPanel.add(new JLabel());
inputPanel.add(addButton);
JPanel outputPanel = new JPanel(new BorderLayout());
outputArea = new JTextArea();
outputArea.setEditable(false);
JScrollPane scrollPane = new JScrollPane(outputArea);
displayButton = new JButton("Display");
displayButton.addActionListener(this);
saveButton = new JButton("Save to File");
saveButton.addActionListener(this);
JPanel buttonPanel = new JPanel(new GridLayout(1, 2));
buttonPanel.add(displayButton);
buttonPanel.add(saveButton);
outputPanel.add(scrollPane, BorderLayout.CENTER);
outputPanel.add(buttonPanel, BorderLayout.SOUTH);
add(inputPanel);
add(outputPanel);
productList = new ArrayList<Product>();
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
String name = nameField.getText();
double price = Double.parseDouble(priceField.getText());
int number = Integer.parseInt(numberField.getText());
Product product = new Product(name, price, number);
productList.add(product);
outputArea.append("Add Product " + product.getName() + " success!\n");
nameField.setText("");
priceField.setText("");
numberField.setText("");
} else if (e.getSource() == displayButton) {
outputArea.setText("");
for (Product product : productList) {
outputArea.append(product.toString() + "\n");
}
} else if (e.getSource() == saveButton) {
try {
FileWriter writer = new FileWriter("product.txt");
for (Product product : productList) {
writer.write(product.getName() + "," + product.getPrice() + "," + product.getNumber() + "\n");
}
writer.flush();
writer.close();
outputArea.append("File write success.\n");
} catch (IOException ex) {
System.err.println("Error writing file.");
}
}
}
public static void main(String[] args) {
ProductSystem productSystem = new ProductSystem();
productSystem.setVisible(true);
}
}
class Product {
private String name;
private double price;
private int number;
public Product(String name, double price, int number) {
this.name = name;
this.price = price;
this.number = number;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
public int getNumber() {
return number;
}
public String toString() {
return name + " $" + price + " x " + number + " = $" + (price * number);
}
}
```
该类实现了您所需的所有功能,包括添加产品,显示产品列表和将产品列表保存到文件。在构造函数中,设置了应用程序的标题,大小和布局,并创建了所有必要的组件。在 actionPerformed 方法中,实现了所有按钮的事件处理程序,包括添加按钮,显示按钮和保存到文件按钮。还使用了一个 Product 类来表示产品,并在 toString 方法中生成产品的字符串表示形式。
阅读全文
相关推荐








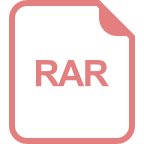








