nested exception is org.apache.ibatis.executor.result.ResultMapException: Error attempting to get column 'in_out' from result set. Cause: java.lang.IllegalArgumentException: No enum constant com.ruoyi.parklot.enums.ParkLot
时间: 2023-10-01 10:07:32 浏览: 267
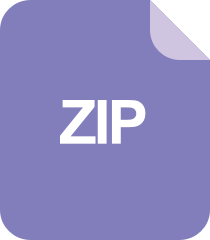
java exception
这个错误是因为 MyBatis 在将查询结果映射为 Java 对象时,无法将数据库中的某些字段值转换为枚举类型。具体地说,这个错误提示中提到的 No enum constant xxx 是因为查询结果中存在某个字段值,在对应的枚举类型中找不到对应的枚举常量。
以您提到的错误提示为例,假设您的查询结果中有一个名为 in_out 的字段,其对应的枚举类型为 ParkLot。在查询结果中,in_out 字段的值为某个字符串,但是在 ParkLot 枚举类型中找不到对应的枚举常量,导致 MyBatis 映射失败,抛出了 ResultMapException 异常。
解决这个问题的方法是,在枚举类型中添加对应的枚举常量。以示例中的 ParkLot 枚举类型为例,可以在其定义中添加一个 UNKNOWN 常量,用于表示查询结果中不在枚举范围内的值:
```java
public enum ParkLot {
IN("1", "入场"),
OUT("2", "出场"),
UNKNOWN("unknown", "未知");
private String value;
private String desc;
ParkLot(String value, String desc) {
this.value = value;
this.desc = desc;
}
public String getValue() {
return value;
}
public String getDesc() {
return desc;
}
public static ParkLot fromValue(String value) {
for (ParkLot item : ParkLot.values()) {
if (item.getValue().equals(value)) {
return item;
}
}
return UNKNOWN;
}
}
```
在枚举类型中,我们添加了一个 UNKNOWN 常量,并在 fromValue 方法中对其进行了处理。如果查询结果中的值在枚举范围内,则返回对应的枚举常量;否则,返回 UNKNOWN 常量。
在查询结果映射时,我们可以使用 MyBatis 的 ResultMap 注解来指定字段值到枚举类型的转换:
```java
@Results(id = "parkLotMap", value = {
@Result(property = "inOut", column = "in_out", javaType = ParkLot.class, typeHandler = EnumTypeHandler.class)
})
public interface CarDao {
@Select("SELECT * FROM car WHERE id = #{id}")
@ResultMap("parkLotMap")
Car findById(Long id);
}
```
在以上的代码中,我们使用了 @Result 注解来指定 in_out 字段对应的枚举类型为 ParkLot,typeHandler 使用了 MyBatis 自带的 EnumTypeHandler,用于将字符串类型转换为枚举类型。这样,在查询结果映射时,MyBatis 就可以自动将 in_out 字段的值转换为对应的 ParkLot 枚举类型。
阅读全文
相关推荐
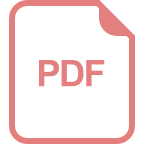
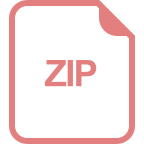


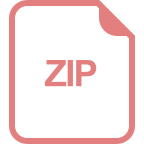
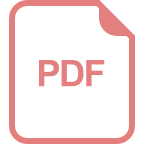
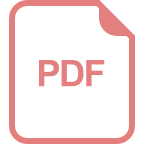
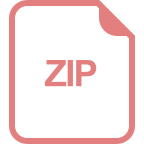
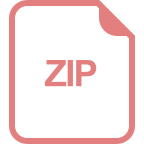
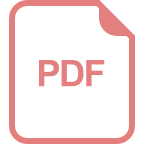
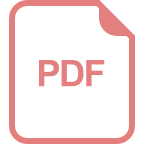
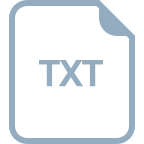
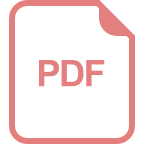
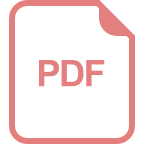
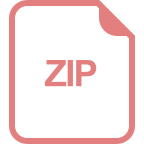