C语言读取wav音频例子
时间: 2023-06-05 18:07:56 浏览: 370
以下是使用C语言读取wav音频的例子:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
char chunkID[4];
int chunkSize;
char format[4];
char subchunk1ID[4];
int subchunk1Size;
short int audioFormat;
short int numChannels;
int sampleRate;
int byteRate;
short int blockAlign;
short int bitsPerSample;
char subchunk2ID[4];
int subchunk2Size;
} WavHeader;
int main() {
FILE* fp;
WavHeader header;
short int* data;
int i;
// 打开wav文件
fp = fopen("example.wav", "rb");
if (fp == NULL) {
printf("File not found!\n");
return -1;
}
// 读取wav文件头
fread(&header, sizeof(WavHeader), 1, fp);
// 打印wav文件信息
printf("Sample rate: %d\n", header.sampleRate);
printf("Channels: %d\n", header.numChannels);
printf("Bits per sample: %d\n", header.bitsPerSample);
printf("Data size: %d bytes\n", header.subchunk2Size);
// 分配内存存储音频数据
data = (short int*)malloc(header.subchunk2Size);
if (data == NULL) {
printf("Memory allocation failed!\n");
return -1;
}
// 读取音频数据
fread(data, header.subchunk2Size, 1, fp);
// 输出前10个采样点的数据
for (i = 0; i < 10; i++) {
printf("%d\n", data[i]);
}
// 关闭文件
fclose(fp);
return 0;
}
```
注意:该例子仅适用于标准的单声道PCM格式的wav音频文件。对于其他格式的音频文件,需要修改代码以正确读取数据。
阅读全文
相关推荐
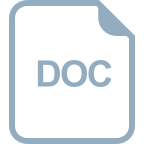
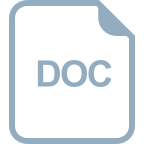
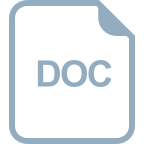





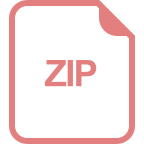
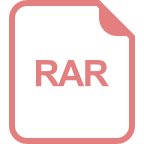
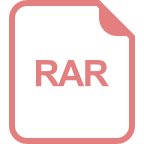
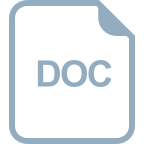
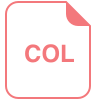





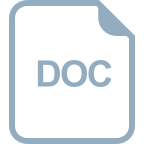