帮我用c语言写一份用蓝牙简易的聊天系统
时间: 2023-10-16 12:06:21 浏览: 76
以下是一个简单的用 C 语言编写的基于蓝牙的聊天系统示例,需要使用到 BlueZ 蓝牙库和 ncurses 界面库:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <bluetooth/bluetooth.h>
#include <bluetooth/rfcomm.h>
#include <ncurses.h>
#define MAX_MESSAGE_LEN 1024
void print_message(const char *message, int is_local) {
attron(COLOR_PAIR(is_local ? 1 : 2));
printw("%s\n", message);
attroff(COLOR_PAIR(is_local ? 1 : 2));
refresh();
}
void send_message(int socket_fd, const char *message) {
if (write(socket_fd, message, strlen(message)) < 0) {
perror("write");
exit(1);
}
print_message(message, 1);
}
void receive_messages(int socket_fd) {
char message[MAX_MESSAGE_LEN];
while (1) {
int bytes_read = read(socket_fd, message, MAX_MESSAGE_LEN - 1);
if (bytes_read < 0) {
perror("read");
exit(1);
} else if (bytes_read == 0) {
break;
}
message[bytes_read] = '\0';
print_message(message, 0);
}
}
int main(int argc, char **argv) {
if (argc < 2) {
fprintf(stderr, "Usage: %s <bluetooth-address>\n", argv[0]);
exit(1);
}
char *server_address_str = argv[1];
// Initialize ncurses
initscr();
start_color();
init_pair(1, COLOR_GREEN, COLOR_BLACK);
init_pair(2, COLOR_MAGENTA, COLOR_BLACK);
clear();
// Create a Bluetooth socket
int socket_fd = socket(AF_BLUETOOTH, SOCK_STREAM, BTPROTO_RFCOMM);
if (socket_fd < 0) {
perror("socket");
exit(1);
}
// Bind the socket to a local Bluetooth address
struct sockaddr_rc local_address = { 0 };
local_address.rc_family = AF_BLUETOOTH;
local_address.rc_bdaddr = *BDADDR_ANY;
local_address.rc_channel = (uint8_t) 1;
if (bind(socket_fd, (struct sockaddr *) &local_address, sizeof(local_address)) < 0) {
perror("bind");
exit(1);
}
// Connect to the remote Bluetooth device
struct sockaddr_rc remote_address = { 0 };
remote_address.rc_family = AF_BLUETOOTH;
str2ba(server_address_str, &remote_address.rc_bdaddr);
remote_address.rc_channel = (uint8_t) 1;
if (connect(socket_fd, (struct sockaddr *) &remote_address, sizeof(remote_address)) < 0) {
perror("connect");
exit(1);
}
// Receive messages in a separate thread
if (fork() == 0) {
receive_messages(socket_fd);
exit(0);
}
// Send messages from the main thread
char message[MAX_MESSAGE_LEN];
while (1) {
getstr(message);
send_message(socket_fd, message);
memset(message, 0, MAX_MESSAGE_LEN);
}
// Close the socket and exit ncurses
close(socket_fd);
endwin();
return 0;
}
```
编译命令:
```
gcc -Wall -o bluetooth_chat bluetooth_chat.c -lbluetooth -lncurses
```
运行命令:
```
./bluetooth_chat <bluetooth-address>
```
其中 `<bluetooth-address>` 是远程蓝牙设备的 MAC 地址。在程序运行后,你可以在终端中输入消息并发送给远程设备,同时也能接收来自远程设备发送的消息。
阅读全文
相关推荐
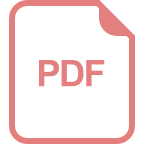

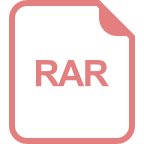
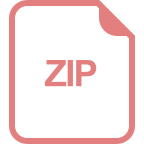
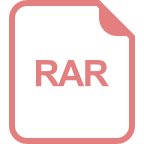
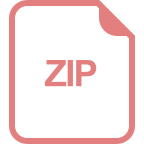
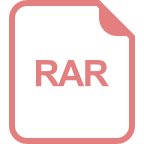
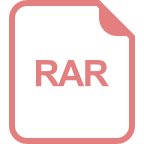
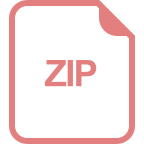