2.Reliability and Maintainability Simulation involves a large number of random variables. The use of dynamic arrays will greatly improve the efficiency of simulation and the scale of problem solving. Please design Vector. This problem requires the impleme
时间: 2024-02-12 08:10:03 浏览: 66
ntation of a dynamic array data structure in C++ that can be used for reliability and maintainability simulation.
Here's an implementation of a Vector class in C++:
```c++
#include <iostream>
#include <stdexcept>
template <typename T>
class Vector {
private:
T* m_data;
size_t m_capacity;
size_t m_size;
public:
Vector() : m_data(nullptr), m_capacity(0), m_size(0) {}
Vector(size_t size) : m_data(new T[size]), m_capacity(size), m_size(size) {}
Vector(const Vector& other) : m_data(new T[other.m_capacity]), m_capacity(other.m_capacity), m_size(other.m_size) {
for (size_t i = 0; i < m_size; ++i) {
m_data[i] = other.m_data[i];
}
}
~Vector() {
delete[] m_data;
}
Vector& operator=(const Vector& other) {
if (this != &other) {
delete[] m_data;
m_data = new T[other.m_capacity];
m_capacity = other.m_capacity;
m_size = other.m_size;
for (size_t i = 0; i < m_size; ++i) {
m_data[i] = other.m_data[i];
}
}
return *this;
}
size_t size() const {
return m_size;
}
size_t capacity() const {
return m_capacity;
}
bool empty() const {
return m_size == 0;
}
void reserve(size_t capacity) {
if (capacity > m_capacity) {
T* temp = m_data;
m_data = new T[capacity];
m_capacity = capacity;
for (size_t i = 0; i < m_size; ++i) {
m_data[i] = temp[i];
}
delete[] temp;
}
}
void resize(size_t size) {
reserve(size);
m_size = size;
}
void push_back(const T& value) {
if (m_capacity == 0) {
reserve(1);
} else if (m_size == m_capacity) {
reserve(2 * m_capacity);
}
m_data[m_size++] = value;
}
void pop_back() {
if (empty()) {
throw std::out_of_range("Vector is empty");
}
--m_size;
}
T& operator[](size_t index) {
if (index >= m_size) {
throw std::out_of_range("Index out of range");
}
return m_data[index];
}
const T& operator[](size_t index) const {
if (index >= m_size) {
throw std::out_of_range("Index out of range");
}
return m_data[index];
}
};
```
This implementation includes methods to get the size, capacity, and emptiness of the vector, as well as methods to reserve space, resize the vector, and add/remove elements at the back. It also provides the [] operator for accessing elements by index.
相关推荐
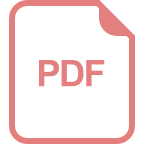
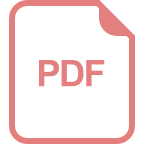
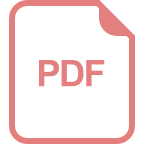

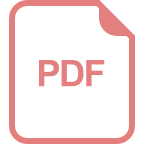
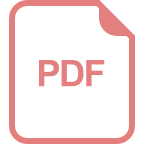
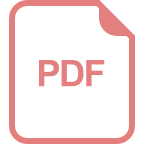
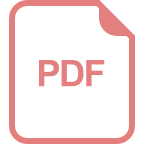
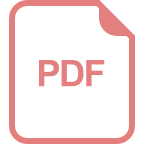
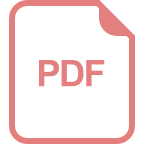
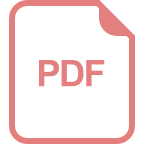
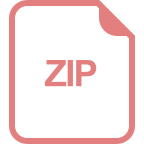
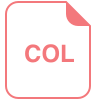

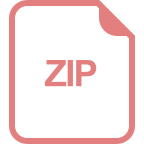
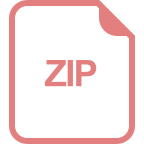