C语言课程设计流星雨
时间: 2025-01-06 16:27:44 浏览: 8
### 关于C语言实现流星雨效果的课程设计
#### 设计概述
为了创建一个基于终端显示的流星雨表白动画,项目需要综合运用多个知识点和技术。这不仅是一个展示个人情感的方式,也是提升编程技能的好机会[^1]。
#### 技术要点分析
- **C语言基础语法**:理解变量声明、控制流语句(if/else, switch)、循环结构(for, while),以及函数定义和调用方法。
- **图形表示与遍历算法**:利用图论中的有向无环图(DAG)模型来构建场景逻辑;采用深度优先搜索(DFS)或广度优先搜索(BFS)来进行路径探索。
- **数据存储方式的选择**:考虑到性能因素,建议选用邻接表作为内部节点连接关系的数据容器。
- **字符界面绘制技术**:掌握如何通过ASCII艺术形式呈现视觉元素,在标准输出设备上模拟动态变化过程。
- **时间管理机制**:引入适当的时间延迟功能,使得整个播放流程更加流畅自然。
#### 示例代码片段
下面给出一段简化版的核心部分伪代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h> // For usleep()
// 定义屏幕大小常量
#define SCREEN_WIDTH 80
#define SCREEN_HEIGHT 25
void clear_screen() {
printf("\e[H\e[J");
}
char screen[SCREEN_HEIGHT][SCREEN_WIDTH];
void init_screen() {
for (int y = 0; y < SCREEN_HEIGHT; ++y)
for (int x = 0; x < SCREEN_WIDTH; ++x)
screen[y][x] = ' ';
}
void draw_star(int x, int y) {
if (x >= 0 && x < SCREEN_WIDTH && y >= 0 && y < SCREEN_HEIGHT)
screen[y][x] = '*';
}
void display_frame() {
clear_screen();
for (int y = 0; y < SCREEN_HEIGHT; ++y) {
for (int x = 0; x < SCREEN_WIDTH; ++x)
putchar(screen[y][x]);
putchar('\n');
}
fflush(stdout);
}
void move_stars_randomly() {
srand(time(NULL));
for (int i = 0; i < 10; ++i) { // 假设有十个星星
int dx = rand() % 3 - 1;
int dy = rand() % 3 - 1;
static struct star_pos pos[] = {{rand()%SCREEN_WIDTH, rand()%SCREEN_HEIGHT}};
char prev_char = screen[pos[i].y][pos[i].x];
screen[pos[i].y][pos[i].x] = ' '; // 清除旧位置
pos[i].x += dx;
pos[i].y += dy;
draw_star(pos[i].x, pos[i].y);
}
}
int main(void){
init_screen();
while(true){
move_stars_randomly();
display_frame();
usleep(100 * 1000); // Delay between frames
}
return EXIT_SUCCESS;
}
```
这段代码展示了基本框架,实际应用中还需要考虑更多细节优化,比如防止越界访问数组等问题,并加入更多的特效以增强观赏性。
阅读全文
相关推荐



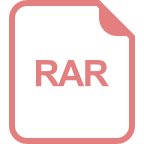
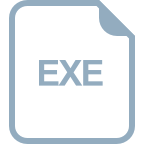


