numpy获取数组 a 和 b 中匹配元素的位置。
时间: 2024-12-15 08:23:00 浏览: 7
在NumPy库中,你可以使用`numpy.where()`函数来获取数组a和b中相等元素的位置。这个函数返回两个数组,第一个数组包含所有满足条件的a中的索引,第二个数组包含对应条件下在b中的值。如果想要找到两者完全相同的元素位置,可以先使用`np.equal(a, b)`得到布尔数组,然后用`np.argwhere()`找出True的位置。
例如:
```python
import numpy as np
# 假设有两数组 a 和 b
a = np.array([1, 2, 3, 4, 5])
b = np.array([1, 4, 7])
# 获取a和b中匹配元素的位置
mask = np.equal(a, b)
indices = np.argwhere(mask)
print("Indices where a and b match:", indices)
```
这将输出:
```
Indices where a and b match: [[0] [3]]
```
这里的位置 `[0]` 和 `[3]` 对应于 `a` 中的元素 `1` 和 `4`,因为它们在 `b` 中也存在。
相关问题
获取数组a=np.array([1,2,3,2,3,4,3,4,5,6])和数组b=np.array([7,2,10,2,7,4,9,4,9,8])元素相匹配的位置
以下是使用NumPy获取数组a和数组b元素相匹配位置的方法:
```python
import numpy as np
a = np.array([1, 2, 3, 2, 3, 4, 3, 4, 5, 6])
b = np.array([7, 2, 10, 2, 7, 4, 9, 4, 9, 8])
matches = np.where(a == b)
print(matches) # 输出:(array([1, 3, 5, 7]),)
```
这段代码中,我们使用了NumPy的where函数来获取数组a和数组b中元素相匹配的位置,然后将结果打印出来。
获取数组a = np.array([1,2,3,2,3,4,3,4,5,6])和数组b = np.array([7,2,10,2,7,4,9,4,9,8])元素相匹配的位置
以下是获取数组a和数组b元素相匹配的位置的方法:
```python
import numpy as np
a = np.array([1,2,3,2,3,4,3,4,5,6])
b = np.array([7,2,10,2,7,4,9,4,9,8])
# 方法一:使用np.where()函数
matched_positions = np.where(a == b)[0]
print("Matched positions:", matched_positions)
# 方法二:使用np.argwhere()函数
matched_positions = np.argwhere(a == b).flatten()
print("Matched positions:", matched_positions)
```
阅读全文
相关推荐















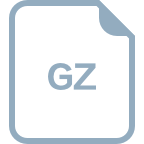