如何使用C语言编程来统计输入字符串中数字、字母和其他符号的数量,并显示其具体内容?
时间: 2024-12-13 07:18:25 浏览: 7
要实现这个功能,你可以按照以下步骤编写C程序:
```c
#include <stdio.h>
#include <ctype.h> // 使用ctype头文件以获得字符分类函数
int main() {
char input[100];
int uppercase_count = 0;
int lowercase_count = 0;
int digit_count = 0;
int other_count = 0;
printf("Enter a string: ");
fgets(input, sizeof(input), stdin); // 从标准输入读取字符串,忽略换行符
for (int i = 0; input[i] != '\0'; i++) { // 遍历字符串
if (isupper(input[i])) { // 判断字符是否为大写
uppercase_count++;
} else if (islower(input[i])) { // 判断字符是否为小写
lowercase_count++;
} else if (isdigit(input[i])) { // 判断字符是否为数字
digit_count++;
} else { // 其他字符(包括空格、标点符号等)
other_count++;
}
}
printf("\nUppercase letters: %d\n", uppercase_count);
printf("Lowercase letters: %d\n", lowercase_count);
printf("Digits: %d\n", digit_count);
printf("Other characters: %d\n", other_count);
return 0;
}
```
该程序首先定义四个计数器分别用于记录大写字母、小写字母、数字和其他字符的数量。接着通过`fgets()`函数读取用户输入的字符串,并遍历每个字符。使用`ctype.h`库中的`isupper()`、`islower()`和`isdigit()`函数判断字符类型。最后,输出各类型的字符数量。
阅读全文
相关推荐
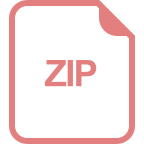
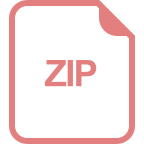

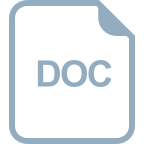













