location.href 发送post请求
时间: 2023-09-08 08:09:01 浏览: 58
你可以使用 JavaScript 中的 `XMLHttpRequest` 对象来发送 POST 请求,并且可以通过设置 `location.href` 实现页面跳转。以下是一个简单的示例:
```javascript
var xhr = new XMLHttpRequest();
xhr.open("POST", "your-url", true);
xhr.setRequestHeader("Content-Type", "application/json");
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
// 请求成功后的操作
location.href = "your-redirect-url";
}
};
var data = {
key1: "value1",
key2: "value2"
};
xhr.send(JSON.stringify(data));
```
在这个示例中,你需要将 `"your-url"` 替换为你要发送 POST 请求的 URL,`"your-redirect-url"` 替换为你要跳转的页面 URL。同时,你可以根据自己的需求修改请求的内容,将 `data` 对象替换为你要发送的数据。
请注意,如果你使用的是现代的 JavaScript 框架(如 React、Vue 等),你可能会使用它们提供的更方便的方式来发送 POST 请求。
相关问题
window.location.href post请求
`window.location.href` 是 JavaScript 中的一个属性,用于获取或设置当前文档的URL。如果你想要发送一个 POST 请求而不是直接使用 `location.href`,你应该使用 `XMLHttpRequest` 或者 `fetch API`。
在JavaScript中,发送POST请求通常不通过`window.location.href`,因为`location.href`主要用于导航,而POST请求是服务器端与客户端数据交换的一种方法,通常是用来提交表单或者进行数据上传。这里是一个使用 `fetch` 发送POST请求的例子:
```javascript
const url = "http://example.com/api/endpoint"; // 替换为你的API地址
const data = { key: 'value' }; // 需要发送的数据
fetch(url, {
method: 'POST', // 设置请求方法为POST
headers: {
'Content-Type': 'application/json' // 根据实际需求设置Content-Type
},
body: JSON.stringify(data), // 将数据转化为JSON字符串并设置为请求体
})
.then(response => response.json()) // 解析响应
.then(data => console.log('Success:', data))
.catch(error => console.error('Error:', error));
```
相关问题:
1. 为什么要使用`fetch`而不是`XMLHttpRequest`发送POST请求?
2. POST请求和GET请求的主要区别是什么?
3. 如何设置`Content-Type`来确保POST数据被正确处理?
location.href post
您可以使用 JavaScript 中的 `location.href` 属性来获取当前页面的 URL。如果您想要通过 POST 方法将数据发送到该 URL,您可以使用 `XMLHttpRequest` 对象或者 `fetch` 函数来实现。以下是一个使用 `XMLHttpRequest` 对象发送 POST 请求的示例代码:
```javascript
var xhr = new XMLHttpRequest();
xhr.open("POST", location.href, true);
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(xhr.responseText);
}
};
xhr.send("data=your_data");
```
请注意,上述代码中的 `"data=your_data"` 是您要发送的数据,您可以根据实际情况进行修改。另外,如果您使用的是服务器端框架,可能需要在服务器端处理 POST 请求并返回相应的结果。
相关推荐
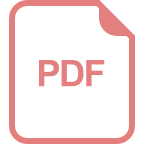
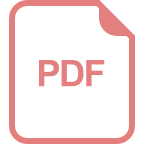
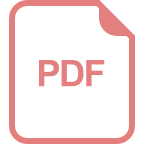













