在,net NAUI中 创建一个自由调整圆角的button
时间: 2024-03-11 10:46:39 浏览: 24
要创建一个自由调整圆角的button,你需要创建一个新控件类并在其中实现自定义行为。
以下是创建自由调整圆角的button的步骤:
1. 创建一个新的控件类,例如MyButton。
```
public class MyButton : Button
{
// ...
}
```
2. 在控件类中添加一个名为CornerRadius的属性,用于设置按钮的圆角半径。
```
public class MyButton : Button
{
public int CornerRadius { get; set; }
// ...
}
```
3. 在控件类的构造函数中设置一些默认值。
```
public class MyButton : Button
{
public int CornerRadius { get; set; }
public MyButton()
{
CornerRadius = 5;
// ...
}
// ...
}
```
4. 在控件类的OnPaint方法中绘制自定义的按钮形状,使用GraphicsPath对象来实现圆角。
```
public class MyButton : Button
{
public int CornerRadius { get; set; }
public MyButton()
{
CornerRadius = 5;
// ...
}
protected override void OnPaint(PaintEventArgs e)
{
GraphicsPath path = new GraphicsPath();
path.AddArc(0, 0, CornerRadius * 2, CornerRadius * 2, 180, 90);
path.AddLine(CornerRadius, 0, Width - CornerRadius, 0);
path.AddArc(Width - CornerRadius * 2, 0, CornerRadius * 2, CornerRadius * 2, 270, 90);
path.AddLine(Width, CornerRadius, Width, Height - CornerRadius);
path.AddArc(Width - CornerRadius * 2, Height - CornerRadius * 2, CornerRadius * 2, CornerRadius * 2, 0, 90);
path.AddLine(Width - CornerRadius, Height, CornerRadius, Height);
path.AddArc(0, Height - CornerRadius * 2, CornerRadius * 2, CornerRadius * 2, 90, 90);
path.AddLine(0, Height - CornerRadius, 0, CornerRadius);
path.CloseFigure();
this.Region = new Region(path);
base.OnPaint(e);
}
}
```
5. 在控件类中重写OnResize方法,以便在控件大小更改时重新绘制按钮形状。
```
public class MyButton : Button
{
public int CornerRadius { get; set; }
public MyButton()
{
CornerRadius = 5;
// ...
}
protected override void OnPaint(PaintEventArgs e)
{
// ...
}
protected override void OnResize(EventArgs e)
{
base.OnResize(e);
this.Invalidate();
}
}
```
现在,你已经可以使用自定义的MyButton控件,并通过设置CornerRadius属性来自由调整圆角半径。
相关推荐
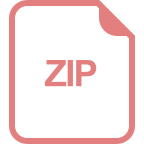





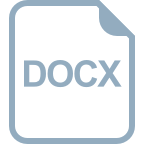
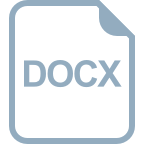
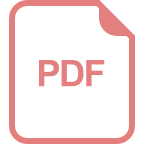