用java写控制流水灯的定时装置,界面可以设定定时时间
时间: 2024-03-21 22:40:34 浏览: 49
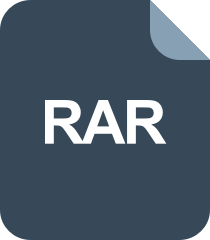
java 写的定时器
好的,这里为您提供一个Java程序,用于控制流水灯的定时装置,并且界面可以设定定时时间。
```java
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.Timer;
public class FlowingLights extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 400;
private static final int HEIGHT = 200;
private static final int DELAY = 1000;
private static final int NUM_LIGHTS = 3;
private static final Color[] COLORS = { Color.RED, Color.GREEN, Color.BLUE };
private JLabel[] lights;
private Timer timer;
private int currentLight = 0;
private boolean isRunning = false;
private int duration = 0;
private JTextField durationField;
public FlowingLights() {
super("Flowing Lights");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(new Dimension(WIDTH, HEIGHT));
setResizable(false);
setLocationRelativeTo(null);
JPanel contentPane = new JPanel();
contentPane.setLayout(new BorderLayout());
JPanel lightsPanel = new JPanel();
lightsPanel.setPreferredSize(new Dimension(400, 100));
lightsPanel.setLayout(new BorderLayout());
lights = new JLabel[NUM_LIGHTS];
for (int i = 0; i < NUM_LIGHTS; i++) {
lights[i] = new JLabel();
lights[i].setPreferredSize(new Dimension(50, 50));
lights[i].setOpaque(true);
lights[i].setBackground(COLORS[i]);
lightsPanel.add(lights[i], i == 0 ? BorderLayout.WEST : i == 1 ? BorderLayout.CENTER : BorderLayout.EAST);
}
JPanel buttonPanel = new JPanel();
buttonPanel.setPreferredSize(new Dimension(400, 50));
JButton timerButton = new JButton("定时");
timerButton.setFont(new Font("宋体", Font.PLAIN, 24));
timerButton.addActionListener(this);
JLabel durationLabel = new JLabel("定时时间(秒):");
durationLabel.setFont(new Font("宋体", Font.PLAIN, 20));
durationField = new JTextField(10);
durationField.setFont(new Font("宋体", Font.PLAIN, 20));
buttonPanel.add(durationLabel);
buttonPanel.add(durationField);
buttonPanel.add(timerButton);
contentPane.add(lightsPanel, BorderLayout.CENTER);
contentPane.add(buttonPanel, BorderLayout.SOUTH);
setContentPane(contentPane);
setVisible(true);
}
public void start() {
isRunning = true;
timer = new Timer(DELAY, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
lights[currentLight].setBackground(COLORS[currentLight]);
currentLight = (currentLight + 1) % NUM_LIGHTS;
lights[currentLight].setBackground(Color.WHITE);
duration--;
if (duration == 0) {
stop();
}
}
});
timer.start();
}
public void stop() {
isRunning = false;
timer.stop();
for (int i = 0; i < NUM_LIGHTS; i++) {
lights[i].setBackground(COLORS[i]);
}
}
@Override
public void actionPerformed(ActionEvent e) {
if (isRunning) {
stop();
} else {
String durationStr = durationField.getText();
if (durationStr.isEmpty()) {
return;
}
duration = Integer.parseInt(durationStr);
start();
}
}
public static void main(String[] args) {
new FlowingLights();
}
}
```
这个程序中,我们添加了一个文本框,用于让用户输入定时时间。当用户点击“定时”按钮时,程序会读取文本框中的值,并开启一个计时器。每当计时器触发一次,程序会切换流水灯的状态,并且将定时时间减少一秒钟。当定时时间为零时,程序会停止运行。
阅读全文
相关推荐


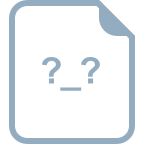
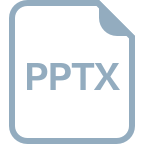
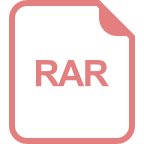
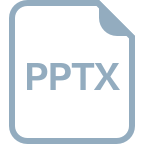
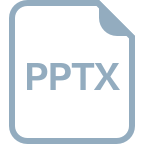
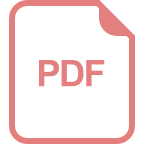
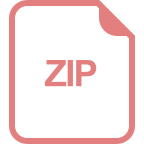
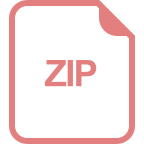
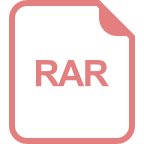
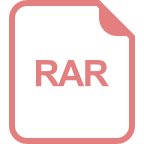
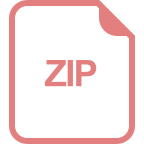