如何实现动态代理
时间: 2024-02-27 17:51:38 浏览: 49
在 Java 中,可以使用 `java.lang.reflect.Proxy` 类和 `java.lang.reflect.InvocationHandler` 接口来实现动态代理。
1. 定义接口:需要被代理的类应该实现一个接口,代理类也应该实现该接口。
2. 实现 InvocationHandler 接口:创建一个类,实现 `InvocationHandler` 接口,该接口有一个方法 `invoke`,在该方法中编写代理逻辑。
3. 使用 Proxy 类创建代理对象:使用 `Proxy.newProxyInstance` 方法创建代理对象,该方法需要传入一个 `ClassLoader` 对象、一个 `Class` 数组和一个 `InvocationHandler` 对象。
示例代码如下:
```java
public interface UserService {
void addUser(String username, String password);
}
public class UserServiceImpl implements UserService {
@Override
public void addUser(String username, String password) {
System.out.println("Add user: " + username + ", password: " + password);
}
}
public class UserServiceProxy implements InvocationHandler {
private Object target;
public UserServiceProxy(Object target) {
this.target = target;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
System.out.println("Before " + method.getName() + " method");
Object result = method.invoke(target, args);
System.out.println("After " + method.getName() + " method");
return result;
}
}
public class Main {
public static void main(String[] args) {
UserService userService = new UserServiceImpl();
UserService proxy = (UserService) Proxy.newProxyInstance(
userService.getClass().getClassLoader(),
userService.getClass().getInterfaces(),
new UserServiceProxy(userService));
proxy.addUser("Alice", "123456");
}
}
```
在上面的示例中,我们定义了一个 `UserService` 接口和一个实现类 `UserServiceImpl`,然后创建了一个 `UserServiceProxy` 类来实现 `InvocationHandler` 接口,该类中实现了代理逻辑,即在方法调用前后打印日志。最后在 `Main` 类中,使用 `Proxy.newProxyInstance` 方法创建了一个代理对象,并调用了代理对象的 `addUser` 方法。运行该程序,输出结果如下:
```
Before addUser method
Add user: Alice, password: 123456
After addUser method
```
从输出结果可以看出,在调用 `addUser` 方法前后,代理类成功地添加了日志记录的逻辑。
阅读全文
相关推荐
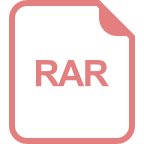
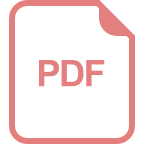
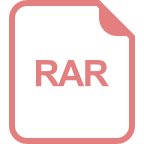
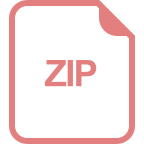
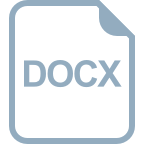
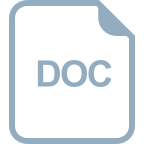
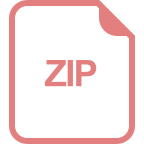
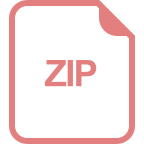
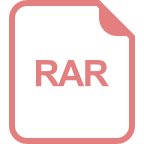
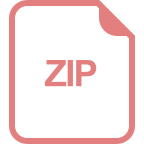
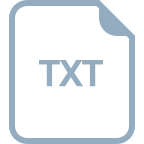
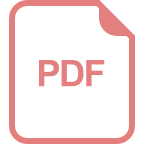
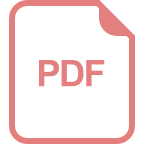
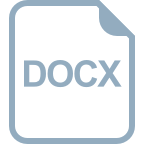