在MFC++6.0中用鼠标选择圆心和半径实现椭圆的扫描转换的代码
时间: 2023-06-19 18:08:37 浏览: 127
以下是一个简单的MFC++ 6.0示例程序,可以使用鼠标选择椭圆的中心和半径,并使用扫描转换算法绘制椭圆:
```cpp
// EllipseScanConversionDlg.h
#if !defined(AFX_ELLIPSESCANCONVERSIONDLG_H__E7BD3A2A_6A5E_4D8F_AE82_8F1B44C55D98__INCLUDED_)
#define AFX_ELLIPSESCANCONVERSIONDLG_H__E7BD3A2A_6A5E_4D8F_AE82_8F1B44C55D98__INCLUDED_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
/////////////////////////////////////////////////////////////////////////////
// CEllipseScanConversionDlg dialog
class CEllipseScanConversionDlg : public CDialog
{
// Construction
public:
CEllipseScanConversionDlg(CWnd* pParent = NULL); // standard constructor
// Dialog Data
//{{AFX_DATA(CEllipseScanConversionDlg)
enum { IDD = IDD_ELLIPSESCANCONVERSION_DIALOG };
//}}AFX_DATA
// ClassWizard generated virtual function overrides
//{{AFX_VIRTUAL(CEllipseScanConversionDlg)
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support
//}}AFX_VIRTUAL
// Implementation
protected:
HICON m_hIcon;
int m_nXCenter, m_nYCenter, m_nXRadius, m_nYRadius;
// Generated message map functions
//{{AFX_MSG(CEllipseScanConversionDlg)
virtual BOOL OnInitDialog();
afx_msg void OnPaint();
afx_msg HCURSOR OnQueryDragIcon();
afx_msg void OnLButtonDown(UINT nFlags, CPoint point);
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
//{{AFX_INSERT_LOCATION}}
// Microsoft Visual C++ will insert additional declarations immediately before the previous line.
#endif // !defined(AFX_ELLIPSESCANCONVERSIONDLG_H__E7BD3A2A_6A5E_4D8F_AE82_8F1B44C55D98__INCLUDED_)
```
```cpp
// EllipseScanConversionDlg.cpp
#include "stdafx.h"
#include "EllipseScanConversion.h"
#include "EllipseScanConversionDlg.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CEllipseScanConversionDlg dialog
CEllipseScanConversionDlg::CEllipseScanConversionDlg(CWnd* pParent /*=NULL*/)
: CDialog(CEllipseScanConversionDlg::IDD, pParent)
{
//{{AFX_DATA_INIT(CEllipseScanConversionDlg)
//}}AFX_DATA_INIT
m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);
m_nXCenter = m_nYCenter = m_nXRadius = m_nYRadius = 0;
}
void CEllipseScanConversionDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CEllipseScanConversionDlg)
//}}AFX_DATA_MAP
}
BEGIN_MESSAGE_MAP(CEllipseScanConversionDlg, CDialog)
//{{AFX_MSG_MAP(CEllipseScanConversionDlg)
ON_WM_PAINT()
ON_WM_QUERYDRAGICON()
ON_WM_LBUTTONDOWN()
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CEllipseScanConversionDlg message handlers
BOOL CEllipseScanConversionDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
return TRUE; // return TRUE unless you set the focus to a control
}
void CEllipseScanConversionDlg::OnPaint()
{
CPaintDC dc(this); // device context for painting
// TODO: Add your message handler code here
if (m_nXRadius > 0 && m_nYRadius > 0)
{
int x, y;
double dAngle;
const double PI = 3.1415926536;
const double dStep = PI / 180.0;
CPoint ptCenter(m_nXCenter, m_nYCenter);
for (int i = 0; i < 360; i++)
{
dAngle = i * dStep;
x = m_nXRadius * cos(dAngle) + 0.5;
y = m_nYRadius * sin(dAngle) + 0.5;
dc.SetPixel(ptCenter.x + x, ptCenter.y + y, RGB(255, 0, 0));
}
}
}
HCURSOR CEllipseScanConversionDlg::OnQueryDragIcon()
{
return (HCURSOR) m_hIcon;
}
void CEllipseScanConversionDlg::OnLButtonDown(UINT nFlags, CPoint point)
{
// TODO: Add your message handler code here and/or call default
m_nXCenter = point.x;
m_nYCenter = point.y;
m_nXRadius = m_nYRadius = 0;
Invalidate();
CDialog::OnLButtonDown(nFlags, point);
}
```
在这个示例程序中,我们使用`OnLButtonDown`处理程序来记录鼠标单击事件的位置,并使用`OnPaint`处理程序来绘制椭圆。 在`OnPaint`处理程序中,我们使用扫描转换算法来绘制椭圆的每个像素。 您可以更改此示例程序以适应您的需求。
阅读全文
相关推荐



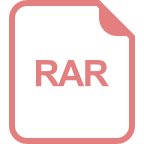
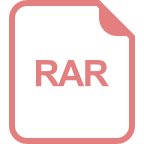



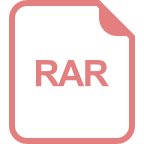


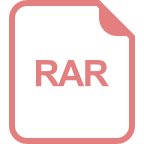
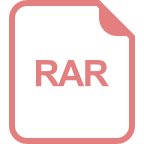





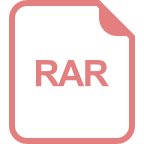