用pyqt5和jedi库实现支持自动补全的python IDE
时间: 2024-05-10 16:16:18 浏览: 11
实现支持自动补全的Python IDE,可以借助PyQt5和Jedi库。
PyQt5是一个Python的GUI框架,可以用来创建各种不同的GUI应用程序,Jedi是一个Python库,用于自动补全、函数签名和代码分析。
以下是一些步骤来实现支持自动补全的Python IDE:
1.安装PyQt5和Jedi库:
```
pip install PyQt5
pip install jedi
```
2.创建一个主窗口,并添加一个代码编辑器和一个自动补全控件:
```python
from PyQt5.QtWidgets import QMainWindow, QTextEdit, QDockWidget, QListWidget
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建代码编辑器
self.editor = QTextEdit()
self.setCentralWidget(self.editor)
# 创建自动补全控件
self.completer = QListWidget()
self.completer.setWindowFlags(Qt.Popup)
self.completer.hide()
self.completer.currentRowChanged.connect(self.insert_completion)
dock = QDockWidget('Autocomplete', self)
dock.setWidget(self.completer)
self.addDockWidget(Qt.RightDockWidgetArea, dock)
```
3.监听代码编辑器的文本变化事件,并在每次文本变化时更新自动补全控件的内容:
```python
from PyQt5.QtCore import Qt, QEvent
class TextEdit(QTextEdit):
def __init__(self, parent=None):
super().__init__(parent)
def event(self, event):
if event.type() == QEvent.KeyPress and event.key() == Qt.Key_Tab:
self.parent().complete()
return True
return super().event(event)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建代码编辑器
self.editor = TextEdit()
self.setCentralWidget(self.editor)
# 创建自动补全控件
self.completer = QListWidget()
self.completer.setWindowFlags(Qt.Popup)
self.completer.hide()
self.completer.currentRowChanged.connect(self.insert_completion)
dock = QDockWidget('Autocomplete', self)
dock.setWidget(self.completer)
self.addDockWidget(Qt.RightDockWidgetArea, dock)
# 监听文本变化事件
self.editor.textChanged.connect(self.update_completer)
```
4.使用Jedi库进行自动补全:
```python
import jedi
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建代码编辑器
self.editor = TextEdit()
self.setCentralWidget(self.editor)
# 创建自动补全控件
self.completer = QListWidget()
self.completer.setWindowFlags(Qt.Popup)
self.completer.hide()
self.completer.currentRowChanged.connect(self.insert_completion)
dock = QDockWidget('Autocomplete', self)
dock.setWidget(self.completer)
self.addDockWidget(Qt.RightDockWidgetArea, dock)
# 监听文本变化事件
self.editor.textChanged.connect(self.update_completer)
def update_completer(self):
# 获取当前光标位置
cursor = self.editor.textCursor()
pos = cursor.position()
# 获取代码内容
code = self.editor.toPlainText()
# 获取当前行的代码
current_line = code.split('\n')[cursor.blockNumber()]
# 获取当前行的所有单词
current_words = current_line[:pos].split()[-1]
# 获取自动补全的内容
completions = jedi.Script(code, path='example.py', line=cursor.blockNumber()+1, column=len(current_words)).completions()
# 显示自动补全内容
self.completer.clear()
for c in completions:
self.completer.addItem(c.name)
if completions:
self.completer.setCurrentRow(0)
self.completer.show()
else:
self.completer.hide()
def complete(self):
# 获取当前光标位置
cursor = self.editor.textCursor()
pos = cursor.position()
# 获取代码内容
code = self.editor.toPlainText()
# 获取当前行的代码
current_line = code.split('\n')[cursor.blockNumber()]
# 获取当前行的所有单词
current_words = current_line[:pos].split()[-1]
# 获取自动补全的内容
completions = jedi.Script(code, path='example.py', line=cursor.blockNumber()+1, column=len(current_words)).completions()
# 插入自动补全内容
if completions:
self.insert_completion(completions[0])
def insert_completion(self, completion):
# 获取当前光标位置
cursor = self.editor.textCursor()
# 获取代码内容
code = self.editor.toPlainText()
# 获取当前行的代码
current_line = code.split('\n')[cursor.blockNumber()]
# 获取当前行的所有单词
current_words = current_line[:cursor.positionInBlock()].split()[-1]
# 计算需要替换的文本
replace_text = completion.text[len(current_words):]
# 替换文本
cursor.movePosition(QTextCursor.Left)
cursor.movePosition(QTextCursor.EndOfWord)
cursor.insertText(replace_text)
```
相关推荐
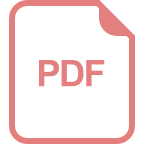
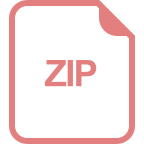














