任务描述 定义一个实数计算类Real,实现浮点数+、-、*、/基本算术运算。 要求:可以进行数据范围[−3.4∗1038,3.4∗1038]检查,数据溢出时显示错误信息。 输入包含多组测试数据,每组输入数据格式:浮点数1 运算符 浮点
时间: 2024-03-02 16:52:01 浏览: 15
数2,其中运算符为+、-、*、/之一。每组数据输出一行,为计算结果或错误信息。
以下是示例代码实现:
```python
class Real:
def __init__(self, num):
self.num = num
def __add__(self, other):
result = self.num + other.num
if abs(result) > 3.4 * 10 ** 38:
return "Error: Data Overflow"
else:
return result
def __sub__(self, other):
result = self.num - other.num
if abs(result) > 3.4 * 10 ** 38:
return "Error: Data Overflow"
else:
return result
def __mul__(self, other):
result = self.num * other.num
if abs(result) > 3.4 * 10 ** 38:
return "Error: Data Overflow"
else:
return result
def __truediv__(self, other):
if other.num == 0:
return "Error: Divide by Zero"
else:
result = self.num / other.num
if abs(result) > 3.4 * 10 ** 38:
return "Error: Data Overflow"
else:
return result
while True:
try:
a, op, b = input().split()
a = Real(float(a))
b = Real(float(b))
if op == '+':
print(a + b)
elif op == '-':
print(a - b)
elif op == '*':
print(a * b)
elif op == '/':
print(a / b)
except EOFError:
break
```
注意,上述代码使用了Python重载运算符的特性,重载了加、减、乘、除四种运算符,实现了浮点数的基本算术运算。同时,为了检查数据范围和溢出情况,使用了Python内置的abs()函数来取绝对值,防止负数的情况。在除法运算时,需要判断除数是否为0,避免出现除以0的错误。最后,使用了Python的异常处理机制,能够在输入结束时自动结束程序。
相关推荐
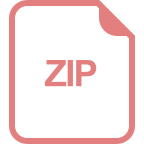
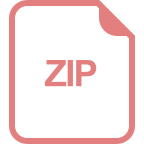














