springboot +vue+elupload图片回显
时间: 2023-10-02 18:13:54 浏览: 123
要实现Spring Boot + Vue + ElementUI 的图片回显功能,需要以下步骤:
1. 在Vue中使用ElementUI的Upload组件上传图片,并将上传成功后的图片地址保存在Vue的data中。
2. 在Spring Boot的Controller中编写接收图片上传请求的方法,将上传的图片保存到服务器上,并返回图片的访问地址。
3. 在Vue中使用img标签展示上传成功的图片,将img标签的src属性设置为上传成功后返回的图片地址。
下面是一个简单的示例代码:
Vue代码:
```
<template>
<div>
<el-upload
class="upload-demo"
:action="uploadUrl"
:on-success="handleUploadSuccess"
:show-file-list="false">
<el-button slot="trigger" size="small" type="primary">点击上传</el-button>
</el-upload>
<img v-if="imageUrl" :src="imageUrl" />
</div>
</template>
<script>
export default {
data() {
return {
imageUrl: '',
uploadUrl: 'http://localhost:8080/upload'
}
},
methods: {
handleUploadSuccess(response) {
this.imageUrl = response.data.url;
}
}
}
</script>
```
Spring Boot代码:
```
@RestController
public class UploadController {
@PostMapping("/upload")
public ResponseEntity<String> upload(@RequestParam("file") MultipartFile file) {
try {
// 保存上传的文件到服务器上
String fileName = file.getOriginalFilename();
String filePath = "path/to/save/" + fileName;
File dest = new File(filePath);
file.transferTo(dest);
// 返回图片的访问地址
String imageUrl = "http://localhost:8080/images/" + fileName;
return ResponseEntity.ok(imageUrl);
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
}
```
注意:上述代码仅供参考,具体实现需要根据自己的需求进行适当的修改。
阅读全文
相关推荐
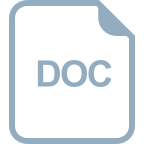
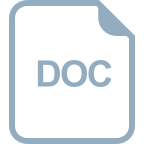
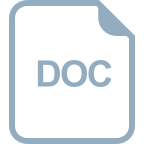
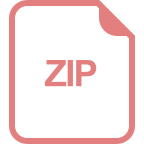
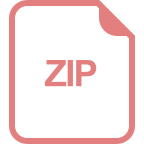
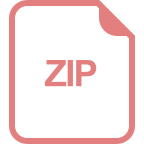
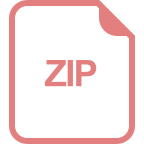
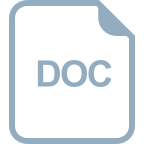
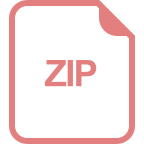
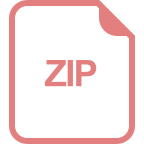
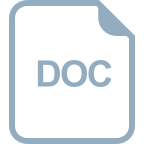
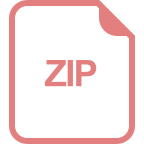
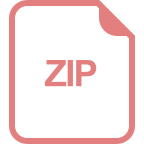
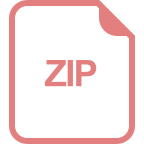
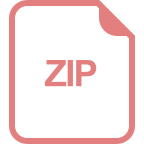
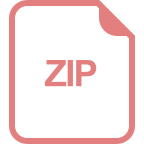
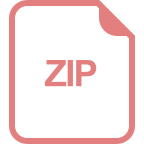
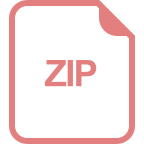
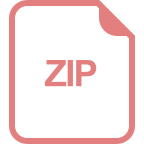