pyqt5 QLable 背景有黑到白变化的动画
时间: 2023-08-22 10:09:40 浏览: 38
要实现 QLabel 背景有黑到白变化的动画效果,可以使用 QTimer 和 QPropertyAnimation 类来控制 QLabel 的背景色动画。
具体步骤如下:
1. 创建一个 QTimer 对象,用于定时触发背景色动画。
```
timer = QTimer()
```
2. 创建一个 QPropertyAnimation 对象,用于控制 QLabel 的背景色动画。
```
animation = QPropertyAnimation(label, b"styleSheet")
```
其中,`label` 表示要进行动画的 QLabel 对象,`b"styleSheet"` 表示对 QLabel 的样式表属性进行动画。
3. 设置 QTimer 的定时器间隔和启动动画。
```
timer.setInterval(2000)
timer.timeout.connect(animation.start)
timer.start()
```
其中,`setInterval` 方法设置定时器的间隔时间,`timeout` 信号连接到 `start` 方法,表示定时器超时时启动动画。
4. 设置 QPropertyAnimation 的动画属性和值。
```
animation.setDuration(1000)
animation.setStartValue("background-color: #000000")
animation.setEndValue("background-color: #FFFFFF")
```
其中,`setDuration` 方法设置动画的持续时间,`setStartValue` 方法设置动画的起始样式表,`setEndValue` 方法设置动画的结束样式表。
5. 在动画结束时,更新 QLabel 的样式表。
```
animation.finished.connect(lambda: label.setStyleSheet("background-color: #FFFFFF"))
```
其中,`finished` 信号连接到一个 lambda 函数,该函数在动画结束时更新 QLabel 的样式表。
最终的代码如下:
```
from PyQt5.QtCore import QTimer, QPropertyAnimation
from PyQt5.QtWidgets import QApplication, QLabel
app = QApplication([])
label = QLabel("Hello, World!")
label.setStyleSheet("background-color: #000000; border: 2px solid #cccccc; border-radius: 10px;")
timer = QTimer()
animation = QPropertyAnimation(label, b"styleSheet")
animation.setDuration(1000)
animation.setStartValue("background-color: #000000")
animation.setEndValue("background-color: #FFFFFF")
animation.finished.connect(lambda: label.setStyleSheet("background-color: #FFFFFF"))
timer.setInterval(2000)
timer.timeout.connect(animation.start)
timer.start()
label.show()
app.exec_()
```
以上代码会显示一个带有黑到白背景色变化动画效果的 QLabel 控件。每隔 2 秒钟,控件的背景色会从黑色变化到白色,并在变化结束时更新样式表。
相关推荐
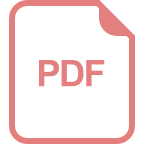














