用自定义模型的方式写出LeNet,不使用Sequential模型,不使用tensorflow
时间: 2024-05-30 22:15:19 浏览: 144
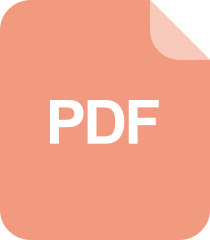
TensorFlow2.X使用图片制作简单的数据集训练模型
或torch框架,你有什么建议?
很高兴能够回答您的问题。如果您想以自定义模型的方式编写LeNet,而不使用Sequential模型,也不使用TensorFlow或Torch框架,我会建议您使用Python中的NumPy库。NumPy是一个十分功能强大的Python库,可以很好地处理矩阵和数组等数学运算。
下面是实现LeNet的一些简要步骤:
1.导入NumPy库。
import numpy as np
2.初始化LeNet的权重和偏置。
W1 = np.random.randn(5, 5, 1, 6)
b1 = np.zeros((6, 1))
W2 = np.random.randn(5, 5, 6, 16)
b2 = np.zeros((16, 1))
W3 = np.random.randn(400, 120)
b3 = np.zeros((120, 1))
W4 = np.random.randn(120, 84)
b4 = np.zeros((84, 1))
W5 = np.random.randn(84, 10)
b5 = np.zeros((10, 1))
3.定义卷积函数。
def convolve(image, kernel, bias, stride = 1, padding = 0):
(iH, iW) = image.shape
(kH, kW) = kernel.shape
oH = int((iH - kH + 2 * padding) / stride) + 1
oW = int((iW - kW + 2 * padding) / stride) + 1
output = np.zeros((oH, oW))
image = np.pad(image, ((padding, padding), (padding, padding)), mode='constant', constant_values=0)
for y in np.arange(padding, iH + padding, stride):
for x in np.arange(padding, iW + padding, stride):
roi = image[y - padding:y + kH - padding, x - padding:x + kW - padding]
output[int((y - padding) / stride), int((x - padding) / stride)] = np.sum(roi * kernel) + bias
return output
4.定义池化函数。
def max_pool(image, kernelSize, stride):
(iH, iW) = image.shape
oH = int((iH - kernelSize) / stride) + 1
oW = int((iW - kernelSize) / stride) + 1
output = np.zeros((oH, oW))
for y in np.arange(0, iH, stride):
for x in np.arange(0, iW, stride):
roi = image[y:y + kernelSize, x:x + kernelSize]
output[int(y / stride), int(x / stride)] = np.max(roi)
return output
5.定义前向传播函数。
def forward(image):
conv1 = convolve(image, W1, b1)
pool1 = max_pool(conv1, 2, 2)
conv2 = convolve(pool1, W2, b2)
pool2 = max_pool(conv2, 2, 2)
flatten = pool2.flatten()
hidden3 = np.dot(W3, flatten) + b3
activate3 = np.maximum(hidden3, 0)
hidden4 = np.dot(W4, activate3) + b4
activate4 = np.maximum(hidden4, 0)
output = np.dot(W5, activate4) + b5
return output
以上代码仅为参考,您可以根据具体情况进行修改,以适应您的数据集和模型框架。希望我的回答能够对您有所帮助。
阅读全文
相关推荐
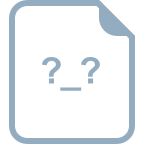
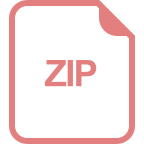


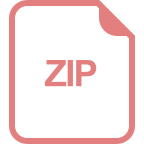
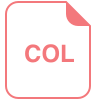
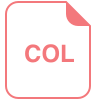
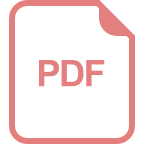
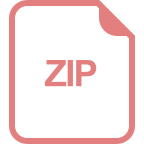
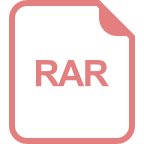
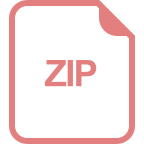
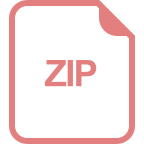
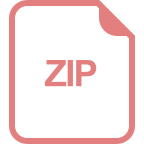
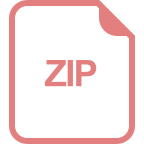
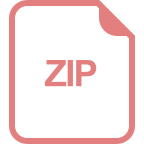
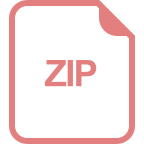
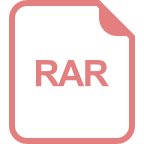