用C语言设计一个程序,输入两个字符串,一长一短,使得短的字符串循环延长至和长的字符串中字母数量相同的长度,要求使用函数和指针数组,若长的字符串中出现空格,短的字符串循环延长时需要跳过
时间: 2023-07-16 10:16:48 浏览: 146
程序如下:
```
#include <stdio.h>
#include <string.h>
void extendString(char *longStr, char *shortStr) {
int longLen = strlen(longStr);
int shortLen = strlen(shortStr);
char *temp = shortStr;
while (*temp) {
if (*temp == ' ') {
shortLen--;
}
temp++;
}
int diff = longLen - shortLen;
if (diff <= 0) {
return;
}
char *ptr[shortLen];
int i;
for (i = 0; i < shortLen; i++) {
ptr[i] = &shortStr[i];
}
temp = shortStr;
for (i = 0; i < diff; i++) {
if (*temp == ' ') {
i--;
} else {
*ptr[i % shortLen] = *temp;
ptr[i % shortLen]++;
}
temp++;
}
}
int main() {
char longStr[100], shortStr[100];
printf("Enter the long string: ");
gets(longStr);
printf("Enter the short string: ");
gets(shortStr);
extendString(longStr, shortStr);
printf("Extended string: %s", shortStr);
return 0;
}
```
使用函数和指针数组,首先计算出长字符串和短字符串的长度差diff。如果diff小于等于0,短字符串就足够长了,无需处理。否则,创建一个指针数组,每个指针指向短字符串中的一个字符。然后遍历短字符串,如果当前字符是空格,就不用管;否则,将指向短字符串中对应字符的指针指向当前字符,然后将这个指针往后移动一个位置。如果短字符串的指针数组满了,就从头开始,直到长字符串和短字符串的长度差为0为止。最后,短字符串就延长到了和长字符串一样长,其中空格被跳过。
阅读全文
相关推荐
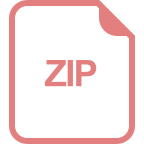
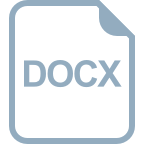
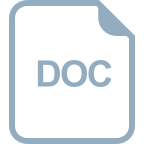







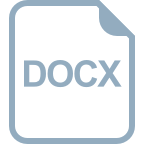
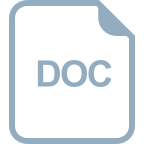
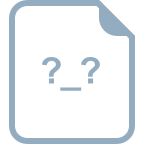
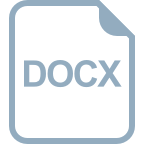
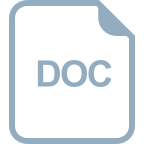
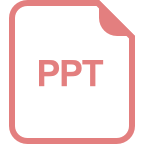
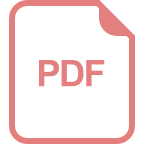
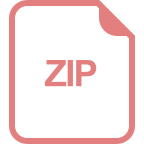