怎么用 C++ 编写一个复数计算器?包括加减乘除、幂次方、取模、辐角、共轭。实部和虚部都是实数范围。
时间: 2023-06-16 14:02:15 浏览: 167
以下是一个简单的复数计算器的 C++ 代码:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0) {
real = r;
imag = i;
}
Complex operator+(Complex const &obj) {
return Complex(real + obj.real, imag + obj.imag);
}
Complex operator-(Complex const &obj) {
return Complex(real - obj.real, imag - obj.imag);
}
Complex operator*(Complex const &obj) {
return Complex(real*obj.real - imag*obj.imag, real*obj.imag + imag*obj.real);
}
Complex operator/(Complex const &obj) {
double den = obj.real*obj.real + obj.imag*obj.imag;
return Complex((real*obj.real + imag*obj.imag) / den, (imag*obj.real - real*obj.imag) / den);
}
Complex operator^(int n) {
double r = sqrt(real*real + imag*imag);
double theta = atan2(imag, real);
return Complex(pow(r, n)*cos(n*theta), pow(r, n)*sin(n*theta));
}
double mod() {
return sqrt(real*real + imag*imag);
}
double arg() {
return atan2(imag, real);
}
Complex conj() {
return Complex(real, -imag);
}
void display() {
cout << real << " + " << imag << "i" << endl;
}
};
int main() {
Complex c1(2, 3);
Complex c2(4, -5);
Complex c3 = c1 + c2;
c3.display();
Complex c4 = c1 - c2;
c4.display();
Complex c5 = c1 * c2;
c5.display();
Complex c6 = c1 / c2;
c6.display();
Complex c7 = c1 ^ 3;
c7.display();
double mod = c1.mod();
cout << "Modulus of c1: " << mod << endl;
double arg = c1.arg();
cout << "Argument of c1: " << arg << endl;
Complex c8 = c1.conj();
c8.display();
return 0;
}
```
在这个实现中,我们定义了一个名为 `Complex` 的类,它有两个私有变量 `real` 和 `imag`,分别表示复数的实部和虚部。我们使用了运算符重载的技术来实现加减乘除、幂次方等操作。同时,我们也提供了计算模、辐角和共轭的函数。在 `main` 函数中,我们创建了两个复数 `c1` 和 `c2`,并进行了加减乘除、幂次方等操作,并输出了结果。
阅读全文
相关推荐


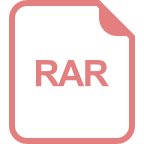













