Iterable实现斐波那契数列
时间: 2023-10-19 12:05:39 浏览: 53
Iterable是一个接口,用于表示可迭代对象。要实现斐波那契数列的迭代器,我们需要实现Iterable接口,并重写其iterator()方法,返回一个实现了Iterator接口的迭代器对象。
以下是一个实现斐波那契数列的Iterable示例代码:
```java
import java.util.Iterator;
public class FibonacciIterable implements Iterable<Integer> {
private int limit;
public FibonacciIterable(int limit) {
this.limit = limit;
}
@Override
public Iterator<Integer> iterator() {
return new FibonacciIterator(limit);
}
private class FibonacciIterator implements Iterator<Integer> {
private int current = 0;
private int next = 1;
private int count = 0;
private int limit;
public FibonacciIterator(int limit) {
this.limit = limit;
}
@Override
public boolean hasNext() {
return count < limit;
}
@Override
public Integer next() {
int result = current;
int temp = current + next;
current = next;
next = temp;
count++;
return result;
}
}
}
```
在上面的代码中,FibonacciIterable实现了Iterable接口,并在其iterator()方法中返回了一个实现了Iterator接口的迭代器对象FibonacciIterator。
FibonacciIterator中实现了hasNext()和next()方法,用于判断是否还有下一个元素和获取下一个元素。在next()方法中,我们实现了斐波那契数列的递推公式f(n) = f(n-1) + f(n-2),并更新了当前值和下一个值。
现在,我们可以使用FibonacciIterable来遍历斐波那契数列了:
```java
public static void main(String[] args) {
FibonacciIterable iterable = new FibonacciIterable(10);
for (int i : iterable) {
System.out.print(i + " ");
}
// Output: 0 1 1 2 3 5 8 13 21 34
}
```
相关推荐
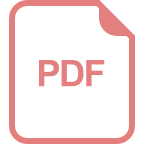
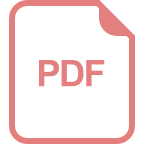








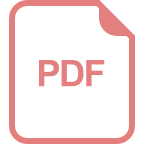
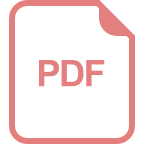
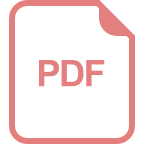
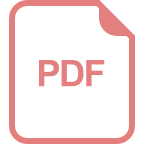
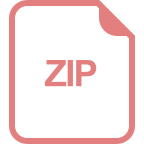
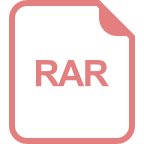