HttpUrlConnection 发送 SOAP 请求,SAX 解析 SOAP 响应
时间: 2024-06-09 07:05:43 浏览: 6
HttpUrlConnection 是 Java 中用来发送 HTTP 请求的类,可以用来发送 SOAP 请求。SOAP 是一种基于 XML 的协议,用于在 Web 上交换数据。在发送 SOAP 请求时,通常需要将请求体中的数据格式化成 XML,然后通过 HttpUrlConnection 发送请求。接下来,我们可以通过 SAX 解析器对响应进行解析。
首先,我们需要构造一个包含 SOAP 请求的 XML 数据。例如,下面是一个简单的 SOAP 请求:
```
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:ser="http://service.example.com/">
<soapenv:Header/>
<soapenv:Body>
<ser:getUser>
<username>john</username>
</ser:getUser>
</soapenv:Body>
</soapenv:Envelope>
```
然后,我们可以使用 HttpUrlConnection 将该请求发送到服务器:
```
URL url = new URL("http://example.com/service");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "text/xml; charset=utf-8");
String xml = "<soapenv:Envelope xmlns:soapenv=\"http://schemas.xmlsoap.org/soap/envelope/\" xmlns:ser=\"http://service.example.com/\"><soapenv:Header/><soapenv:Body><ser:getUser><username>john</username></ser:getUser></soapenv:Body></soapenv:Envelope>";
conn.setDoOutput(true);
OutputStream os = conn.getOutputStream();
os.write(xml.getBytes());
os.flush();
os.close();
```
在发送请求后,我们可以使用 SAX 解析器对响应进行解析。例如,我们可以解析包含用户信息的响应:
```
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:ser="http://service.example.com/">
<soapenv:Header/>
<soapenv:Body>
<ser:getUserResponse>
<user>
<name>John Doe</name>
<age>30</age>
<email>john@example.com</email>
</user>
</ser:getUserResponse>
</soapenv:Body>
</soapenv:Envelope>
```
我们可以使用 SAX 解析器解析用户信息:
```
String responseXml = readResponse(conn);
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser saxParser = factory.newSAXParser();
UserHandler userHandler = new UserHandler();
saxParser.parse(new InputSource(new StringReader(responseXml)), userHandler);
User user = userHandler.getUser();
System.out.println("Name: " + user.getName());
System.out.println("Age: " + user.getAge());
System.out.println("Email: " + user.getEmail());
```
其中,UserHandler 是一个自定义的 SAX 解析器,用于解析用户信息:
```
public class UserHandler extends DefaultHandler {
private User user;
private String currentElement;
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
currentElement = qName;
if ("user".equals(qName)) {
user = new User();
}
}
public void characters(char[] ch, int start, int length) throws SAXException {
String value = new String(ch, start, length).trim();
if ("name".equals(currentElement)) {
user.setName(value);
} else if ("age".equals(currentElement)) {
user.setAge(Integer.parseInt(value));
} else if ("email".equals(currentElement)) {
user.setEmail(value);
}
currentElement = "";
}
public User getUser() {
return user;
}
}
```
User 是一个简单的 Java 类,用于保存用户信息:
```
public class User {
private String name;
private int age;
private String email;
// getter and setter methods
}
```
相关推荐
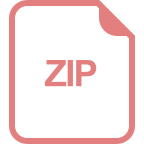
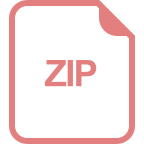














