用c++制作一个五子棋小游戏
时间: 2023-11-27 18:20:48 浏览: 36
好的,以下是一个简单的C++五子棋小游戏代码示例:
```c++
#include <iostream>
#include <vector>
using namespace std;
const int BOARD_SIZE = 15; // 棋盘大小
class ChessBoard {
public:
enum ChessType {
EMPTY = 0,
BLACK,
WHITE
};
ChessBoard() {
for (int i = 0; i < BOARD_SIZE; ++i) {
board_.push_back(vector<ChessType>(BOARD_SIZE, EMPTY));
}
}
bool PutChess(int x, int y, ChessType type) {
if (x < 0 || x >= BOARD_SIZE || y < 0 || y >= BOARD_SIZE || board_[x][y] != EMPTY) {
return false;
}
board_[x][y] = type;
return true;
}
bool IsWin(int x, int y, ChessType type) {
int count = 0;
for (int i = x + 1; i < BOARD_SIZE && board_[i][y] == type; ++i) { // 横向
++count;
}
for (int i = x - 1; i >= 0 && board_[i][y] == type; --i) { // 横向
++count;
}
if (count >= 4) {
return true;
}
count = 0;
for (int i = y + 1; i < BOARD_SIZE && board_[x][i] == type; ++i) { // 纵向
++count;
}
for (int i = y - 1; i >= 0 && board_[x][i] == type; --i) { // 纵向
++count;
}
if (count >= 4) {
return true;
}
count = 0;
for (int i = x + 1, j = y + 1; i < BOARD_SIZE && j < BOARD_SIZE && board_[i][j] == type; ++i, ++j) { // 正对角线
++count;
}
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0 && board_[i][j] == type; --i, --j) { // 正对角线
++count;
}
if (count >= 4) {
return true;
}
count = 0;
for (int i = x + 1, j = y - 1; i < BOARD_SIZE && j >= 0 && board_[i][j] == type; ++i, --j) { // 反对角线
++count;
}
for (int i = x - 1, j = y + 1; i >= 0 && j < BOARD_SIZE && board_[i][j] == type; --i, ++j) { // 反对角线
++count;
}
if (count >= 4) {
return true;
}
return false;
}
void Print() {
for (int i = 0; i < BOARD_SIZE; ++i) {
for (int j = 0; j < BOARD_SIZE; ++j) {
switch (board_[i][j]) {
case EMPTY:
cout << "+ ";
break;
case BLACK:
cout << "X ";
break;
case WHITE:
cout << "O ";
break;
}
}
cout << endl;
}
}
private:
vector<vector<ChessType>> board_;
};
int main() {
ChessBoard board;
ChessBoard::ChessType cur = ChessBoard::BLACK;
int x, y;
while (true) {
cout << "Player " << (cur == ChessBoard::BLACK ? "X" : "O") << " please input the position (x y): ";
cin >> x >> y;
if (board.PutChess(x, y, cur)) {
board.Print();
if (board.IsWin(x, y, cur)) {
cout << "Player " << (cur == ChessBoard::BLACK ? "X" : "O") << " win!" << endl;
break;
}
cur = (cur == ChessBoard::BLACK ? ChessBoard::WHITE : ChessBoard::BLACK);
} else {
cout << "Invalid input!" << endl;
}
}
return 0;
}
```
这个示例代码实现了一个简单的控制台五子棋小游戏。你可以根据自己的需要进行修改和优化。
相关推荐
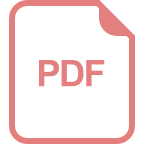
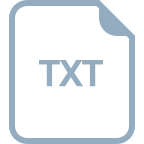
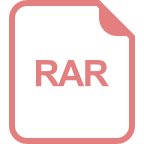














