c语言让屏幕输出居中
时间: 2023-08-16 16:05:17 浏览: 172
你可以使用C语言编写一个函数来实现让屏幕输出居中的功能。以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <windows.h> // 仅限Windows环境
void center_text(char *str)
{
CONSOLE_SCREEN_BUFFER_INFO csbi;
int columns;
GetConsoleScreenBufferInfo(GetStdHandle(STD_OUTPUT_HANDLE), &csbi);
columns = csbi.srWindow.Right - csbi.srWindow.Left + 1;
int len = strlen(str);
int pad = (columns - len) / 2;
printf("%*s%s", pad, "", str);
}
int main()
{
center_text("Hello, world!");
return 0;
}
```
这个函数中,我们首先使用了Windows API函数`GetConsoleScreenBufferInfo`来获取屏幕的宽度,然后计算出需要填充的空格数,最后使用`printf`函数输出字符串并填充空格,就可以实现让屏幕输出居中了。
相关问题
c语言printf输出居中
在C语言中,可以使用printf函数输出居中的字符串。可以使用占位符来控制输出的格式。例如,可以使用%-Ns来控制输出的字符串占用N个字符的宽度,并且左对齐。如果要居中对齐,可以使用%Ns来控制输出的字符串占用N个字符的宽度,并且居中对齐。其中,N是一个整数,表示输出的宽度。具体实现方法如下:
1. 首先计算出字符串的长度len。
2. 然后计算出左侧空格的数量(left_spaces)和右侧空格的数量(right_spaces)。
3. 最后使用printf函数输出字符串,格式为"%*s",其中*表示输出的宽度,s表示输出的字符串。
具体代码如下:
```
void print_center(char *str, int width) {
int len = strlen(str);
int left_spaces = (width - len) / 2;
int right_spaces = width - len - left_spaces;
printf("%*s%s%*s", left_spaces, "", str, right_spaces, "");
}
```
使用示例:
```
print_center("Hello, world!", 20);
```
输出结果为:
```
Hello, world!
```
c语言输出居中
在 C 语言中,可以使用 printf 函数输出居中的文本。可以通过以下代码实现:
```c
#include <stdio.h>
#include <string.h>
int main() {
char text[] = "Hello, World!";
int width = 20; // 输出的总宽度
int spaces = (width - strlen(text)) / 2; // 需要填充的空格数量
// 输出左边的空格
for (int i = 0; i < spaces; i++) {
printf(" ");
}
// 输出文本
printf("%s", text);
// 输出右边的空格
for (int i = 0; i < spaces; i++) {
printf(" ");
}
// 如果需要,输出最后一个空格
if ((width - strlen(text)) % 2 != 0) {
printf(" ");
}
return 0;
}
```
这段代码将输出一个总宽度为 20 的居中文本,例如:
```
Hello, World!
```
相关推荐
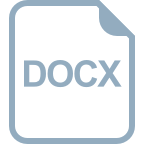
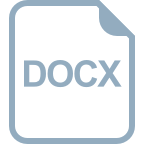












