Java写一个发送邮件,要求带代码生成的附件
时间: 2024-02-19 22:03:17 浏览: 65
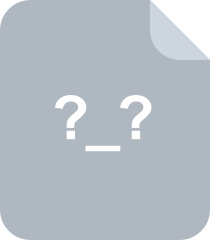
Java实现发送邮件(带附件)功能
好的,以下是Java代码实现发送带代码生成的附件的邮件:
```java
import javax.activation.DataHandler;
import javax.activation.DataSource;
import javax.activation.FileDataSource;
import javax.mail.*;
import javax.mail.internet.*;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
public class SendEmail {
public static void main(String[] args) throws MessagingException, IOException {
// 发件人邮箱地址
String from = "your_email@example.com";
// 发件人邮箱密码或授权码
String password = "your_email_password_or_authorization_code";
// 收件人邮箱地址
String to = "recipient_email@example.com";
// 邮件主题
String subject = "邮件主题";
// 代码文件路径
String codeFilePath = "code_file_path";
// 代码文件名
String codeFileName = "code_file_name";
// 代码文件类型
String codeFileType = "code_file_type";
// 创建邮件会话
Properties props = new Properties();
props.setProperty("mail.transport.protocol", "smtp");
props.setProperty("mail.smtp.host", "smtp.example.com");
props.setProperty("mail.smtp.auth", "true");
Session session = Session.getDefaultInstance(props, new Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(from, password);
}
});
// 创建邮件消息
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress(from));
message.setRecipient(Message.RecipientType.TO, new InternetAddress(to));
message.setSubject(subject);
// 创建代码文件
File codeFile = new File(codeFilePath, codeFileName + "." + codeFileType);
FileWriter fileWriter = new FileWriter(codeFile);
fileWriter.write("public class HelloWorld {\n" +
" public static void main(String[] args) {\n" +
" System.out.println(\"Hello, world!\");\n" +
" }\n" +
"}\n");
fileWriter.close();
// 创建附件
MimeBodyPart attachmentPart = new MimeBodyPart();
DataSource source = new FileDataSource(codeFile);
attachmentPart.setDataHandler(new DataHandler(source));
attachmentPart.setFileName(MimeUtility.encodeText(codeFileName + "." + codeFileType, StandardCharsets.UTF_8.name(), null));
// 创建邮件内容
MimeMultipart multipart = new MimeMultipart();
multipart.addBodyPart(attachmentPart);
message.setContent(multipart);
// 发送邮件
Transport.send(message);
}
}
```
其中,需要替换的参数有:
- `from`: 发件人邮箱地址
- `password`: 发件人邮箱密码或授权码
- `to`: 收件人邮箱地址
- `subject`: 邮件主题
- `codeFilePath`: 代码文件路径
- `codeFileName`: 代码文件名
- `codeFileType`: 代码文件类型
以上代码中,我们先创建了一个代码文件,并将其保存到指定路径下。然后,我们创建一个`MimeBodyPart`对象,将代码文件作为附件添加到邮件中。最后,我们使用`Transport.send()`方法发送邮件。
需要注意的是,我们在设置附件的文件名时,使用了`MimeUtility.encodeText()`方法进行编码,以解决中文文件名乱码的问题。
阅读全文
相关推荐

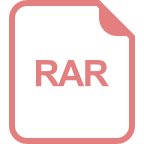
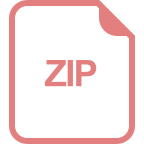
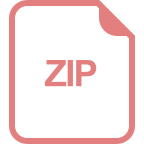
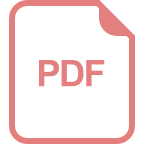
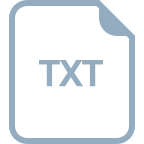
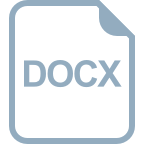
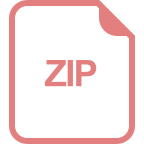
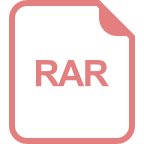
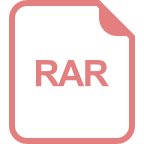
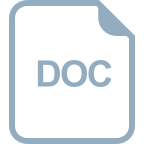
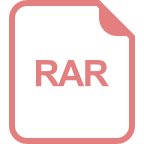
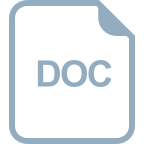


