Java往本地磁盘读写文件的代码
时间: 2024-05-02 08:19:51 浏览: 114
可以使用Java的File类和IO类来进行本地磁盘文件的读写操作。以下是一个简单的示例:
读取文件:
```java
File file = new File("file_name.txt");
try {
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
// 处理读取到的每一行数据
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
```
写入文件:
```java
File file = new File("file_name.txt");
try {
BufferedWriter writer = new BufferedWriter(new FileWriter(file));
writer.write("写入的数据");
writer.newLine(); // 换行
writer.flush();
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
```
注意:需要处理文件不存在或者权限不足的情况,并且文件读写完毕后需要关闭流。
相关问题
使用idea开发环境完成java实现封装读写本地磁盘的xml文件的工具
在使用 IntelliJ IDEA 开发环境完成 Java 实现封装读写本地磁盘的 XML 文件的工具时,你可以按照以下步骤进行:
1. **创建 Java 项目**:
- 打开 IntelliJ IDEA,选择 "Create New Project"。
- 选择 "Java",并根据需要选择合适的 JDK 版本。
- 完成项目创建向导。
2. **添加依赖库**:
- 如果你需要使用 XML 处理库,如 JDOM、DOM4J 或 JAXB,可以在项目的 `pom.xml` 文件中添加相应的 Maven 依赖(如果是 Maven 项目)。例如,添加 JDOM 依赖的代码片段如下:
```xml
<dependency>
<groupId>jdom</groupId>
<artifactId>jdom</artifactId>
<version>2.0.2</version>
</dependency>
```
- 如果是非 Maven 项目,需要手动下载库文件并添加到项目的类路径中。
3. **实现读取 XML 功能**:
- 使用 `FileInputStream` 读取本地磁盘上的 XML 文件。
- 利用 XML 处理库解析读取到的数据。
- 提供一个方法封装整个读取过程,例如:
```java
public Element readXmlFile(String filePath) throws JDOMException, IOException {
SAXBuilder builder = new SAXBuilder();
Document document = builder.build(new File(filePath));
return document.getRootElement();
}
```
4. **实现写入 XML 功能**:
- 创建 XML 结构的文档对象,例如使用 `Document` 和 `Element` 类。
- 利用 XML 处理库的方法构建你想要的 XML 结构。
- 将构建好的 XML 文档写入本地磁盘,可以使用 `XMLOutputter` 类,例如:
```java
public void writeXmlFile(String filePath, Document document) throws IOException {
XMLOutputter xmlOutput = new XMLOutputter();
xmlOutput.output(document, new FileOutputStream(new File(filePath)));
}
```
5. **封装工具类**:
- 将读写 XML 的功能封装在一个工具类中,对外提供简洁的 API。
- 可以包含异常处理逻辑,确保读写操作的健壮性。
6. **测试工具类**:
- 编写测试方法来验证封装好的工具类是否能够正确读写 XML 文件。
java编程完成一个商品销售管理系统,实现了对商品的查询、购买、添加以及删除等操作。 要求: 1、商品信息以文本文件形式存储在本地磁盘中,一行存放一条商品信息,包括商品编号(id)、商品名称(name)、商品价格(price)、商品数量(nums)、上架时间(time)等五个字段,每个字段之间由逗号隔开。 2、使用集合来管理商品,对商品的查询、添加、购买、删除实际上就转变为从集合中查询、添加、删除商品对象。 3、在对商品进行购买、添加、删除等更新操作时,每完成一个更新操作都需要进行一次文件写入,即将最新修改结果实时写入文件内
代码如下:
```java
import java.io.*;
import java.util.ArrayList;
import java.util.Scanner;
public class CommodityManagementSystem {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
ArrayList<Commodity> commodityList = new ArrayList<>();
File file = new File("commodity.txt");
if (!file.exists()) {
try {
file.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
}
try (BufferedReader reader = new BufferedReader(new FileReader(file))) {
String line;
while ((line = reader.readLine()) != null) {
String[] arr = line.split(",");
Commodity commodity = new Commodity(arr[0], arr[1], Double.parseDouble(arr[2]), Integer.parseInt(arr[3]), arr[4]);
commodityList.add(commodity);
}
} catch (IOException e) {
e.printStackTrace();
}
while (true) {
System.out.println("请选择操作:1.查询商品信息 2.购买商品 3.添加新商品 4.删除商品 5.退出系统");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.println("请输入商品编号或名称:");
String keyword = scanner.next();
boolean find = false;
for (Commodity commodity : commodityList) {
if (commodity.getId().equals(keyword) || commodity.getName().equals(keyword)) {
System.out.println(commodity);
find = true;
}
}
if (!find) {
System.out.println("未找到匹配的商品信息!");
}
break;
case 2:
System.out.println("请输入商品编号:");
String id = scanner.next();
Commodity buyCommodity = null;
for (Commodity commodity : commodityList) {
if (commodity.getId().equals(id)) {
buyCommodity = commodity;
break;
}
}
if (buyCommodity == null) {
System.out.println("未找到匹配的商品信息!");
} else {
System.out.println("请输入购买数量:");
int nums = scanner.nextInt();
if (buyCommodity.getNums() < nums) {
System.out.println("库存不足!");
} else {
buyCommodity.setNums(buyCommodity.getNums() - nums);
System.out.println("购买成功!总价为:" + buyCommodity.getPrice() * nums);
try (BufferedWriter writer = new BufferedWriter(new FileWriter(file))) {
for (Commodity commodity : commodityList) {
writer.write(commodity.toString());
writer.newLine();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
break;
case 3:
System.out.println("请输入商品编号、名称、价格、数量、上架时间:");
String newId = scanner.next();
String newName = scanner.next();
double newPrice = scanner.nextDouble();
int newNums = scanner.nextInt();
String newTime = scanner.next();
Commodity newCommodity = new Commodity(newId, newName, newPrice, newNums, newTime);
commodityList.add(newCommodity);
try (BufferedWriter writer = new BufferedWriter(new FileWriter(file))) {
for (Commodity commodity : commodityList) {
writer.write(commodity.toString());
writer.newLine();
}
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("添加成功!");
break;
case 4:
System.out.println("请输入商品编号:");
String deleteId = scanner.next();
boolean deleteSuccess = false;
for (Commodity commodity : commodityList) {
if (commodity.getId().equals(deleteId)) {
commodityList.remove(commodity);
System.out.println("删除成功!");
deleteSuccess = true;
break;
}
}
if (!deleteSuccess) {
System.out.println("未找到匹配的商品信息!");
} else {
try (BufferedWriter writer = new BufferedWriter(new FileWriter(file))) {
for (Commodity commodity : commodityList) {
writer.write(commodity.toString());
writer.newLine();
}
} catch (IOException e) {
e.printStackTrace();
}
}
break;
case 5:
System.out.println("退出系统!");
return;
default:
System.out.println("输入有误,请重新输入!");
}
}
}
}
class Commodity {
private String id;
private String name;
private double price;
private int nums;
private String time;
public Commodity(String id, String name, double price, int nums, String time) {
this.id = id;
this.name = name;
this.price = price;
this.nums = nums;
this.time = time;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getNums() {
return nums;
}
public void setNums(int nums) {
this.nums = nums;
}
public String getTime() {
return time;
}
public void setTime(String time) {
this.time = time;
}
@Override
public String toString() {
return id + "," + name + "," + price + "," + nums + "," + time;
}
}
```
实现思路:
1. 读取文件中的商品信息,将每一行数据按逗号分隔,封装成商品对象,并加入集合中。
2. 根据用户输入的选择,进行查询、购买、添加、删除等操作。
3. 在更新商品信息后,将集合中的最新数据写入文件。
需要注意的点:
1. 文件的读写,使用 try-with-resources 语句,在代码块结束时自动关闭流。
2. 对于输入商品价格和数量等浮点数和整数类型的数据,使用 nextDouble 和 nextInt 方法读取,避免使用 nextLine 方法造成输入错误。
3. 商品信息的存储和读取,使用 toString 和构造方法实现对象的转换。
4. 集合中的对象的删除,不能在遍历集合的过程中直接删除对象,应该使用迭代器进行删除,否则会报 ConcurrentModificationException 异常。
阅读全文
相关推荐
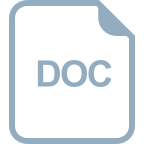
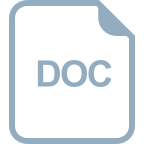
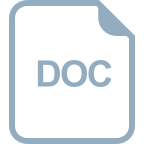
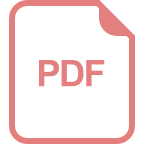
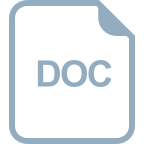
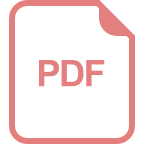
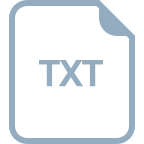
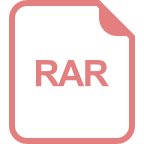
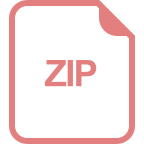
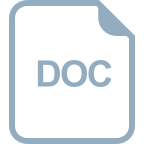
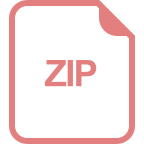
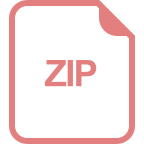
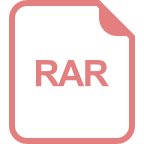
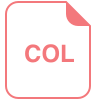
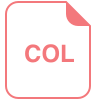
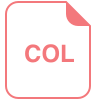
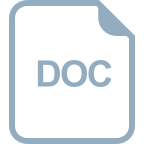