读取xml并转换为Java对象
时间: 2024-01-30 18:03:32 浏览: 129
要将XML数据转换为Java对象,你可以使用Java的内置XML解析器,例如DOM或SAX,或者使用第三方库如Jackson或JAXB。
这里我提供一个使用JAXB的例子,步骤如下:
1. 创建XML文件,并定义对应的Java类。
例如,我们有以下XML文件:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<book>
<title>Java Programming</title>
<author>John Smith</author>
<isbn>1234567890</isbn>
</book>
```
我们可以定义一个名为Book的Java类,如下:
```java
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class Book {
private String title;
private String author;
private String isbn;
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getIsbn() {
return isbn;
}
public void setIsbn(String isbn) {
this.isbn = isbn;
}
}
```
注意,我们需要在Book类上添加@XmlRootElement注解,以指定XML根元素的名称。
2. 使用JAXB将XML文件转换为Java对象。
```java
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Unmarshaller;
import java.io.File;
public class Main {
public static void main(String[] args) {
try {
File file = new File("book.xml");
JAXBContext jaxbContext = JAXBContext.newInstance(Book.class);
Unmarshaller jaxbUnmarshaller = jaxbContext.createUnmarshaller();
Book book = (Book) jaxbUnmarshaller.unmarshal(file);
System.out.println(book.getTitle());
System.out.println(book.getAuthor());
System.out.println(book.getIsbn());
} catch (JAXBException e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们首先创建了一个File对象,用于指定XML文件的位置。然后,我们使用JAXBContext创建了一个Unmarshaller对象,并使用该对象将XML文件转换为Book对象。最后,我们可以访问Book对象的属性并将其打印到控制台上。
以上就是使用JAXB将XML数据转换为Java对象的基本步骤。
阅读全文
相关推荐
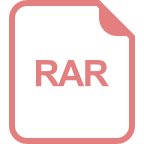
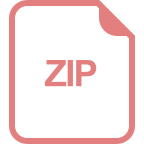
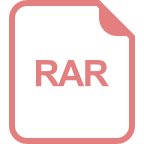
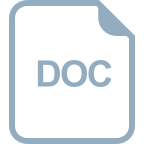
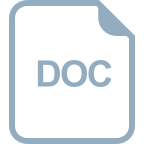
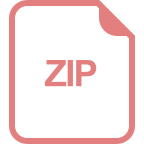
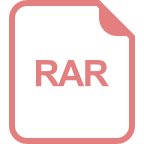
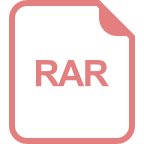
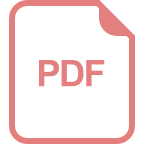
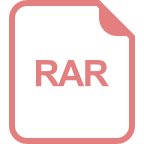
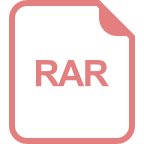
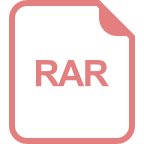

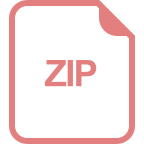
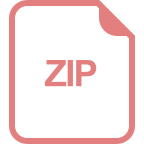
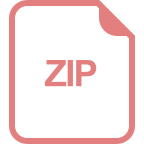
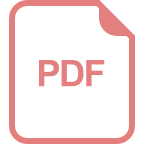