OC语言实现左右翻转uiimage图像
时间: 2024-05-10 07:14:15 浏览: 5
可以通过以下代码实现UIImage图像的左右翻转:
```objc
UIImage *originalImage = [UIImage imageNamed:@"originalImage"]; // 原始图像
UIImage *flippedImage = [UIImage imageWithCGImage:originalImage.CGImage scale:originalImage.scale orientation:UIImageOrientationUpMirrored]; // 左右翻转后的图像
```
其中,UIImageOrientationUpMirrored 表示左右翻转的方向。通过该代码,可以获取到原始图像的左右翻转后的图像 flippedImage。
相关问题
OC左右翻转uiimage图像
可以使用UIImage的`imageFlippedForRightToLeftLayoutDirection`方法来实现UIImage图像的左右翻转,示例代码如下:
```swift
if let image = UIImage(named: "example_image") {
let flippedImage = image.imageFlippedForRightToLeftLayoutDirection()
// 使用flippedImage来显示左右翻转后的图像
}
```
注意:该方法仅在iOS9及以上版本可用。如果您需要在iOS8及以下版本中使用该方法,可以使用以下扩展:
```swift
extension UIImage {
func imageFlippedForRightToLeftLayoutDirectionIfNeeded() -> UIImage {
if #available(iOS 10.0, *) {
return self.imageFlippedForRightToLeftLayoutDirection()
} else {
guard let cgImage = self.cgImage else { return self }
return UIImage(cgImage: cgImage, scale: self.scale, orientation: .upMirrored)
}
}
}
```
然后使用`imageFlippedForRightToLeftLayoutDirectionIfNeeded()`方法来实现左右翻转。
swift怎么左右翻转一个uiimage
可以使用UIImage的`imageFlippedForRightToLeftLayoutDirection`方法来左右翻转UIImage,如下所示:
```swift
if let image = UIImage(named: "yourImageName") {
let flippedImage = image.imageFlippedForRightToLeftLayoutDirection()
// 使用flippedImage进行后续操作
}
```
此方法会根据当前系统的语言环境来确定左右翻转的方向。如果要强制进行左右翻转,可以使用下面的代码:
```swift
if let image = UIImage(named: "yourImageName") {
let flippedImage = UIImage(cgImage: image.cgImage!, scale: image.scale, orientation: .upMirrored)
// 使用flippedImage进行后续操作
}
```
此方法通过创建一个新的UIImage对象,并使用原始UIImage的CGImage和缩放比例,同时使用`.upMirrored`选项来左右翻转图像。
相关推荐
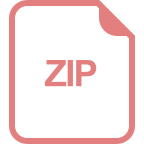
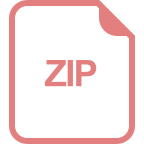
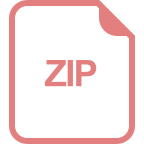












