private void timer1_Tick(object sender, EventArgs e) { if (totalTime >= 20000)// 最多读20秒 { timer1.Stop(); ShutCamera(); textBox1.Text = "未识别到二维码"; return; } Bitmap picture; picture = videoSourcePlayer1.GetCurrentVideoFrame();//拍摄 if (picture != null) { // 识读QR码 BarcodeReader reader = new BarcodeReader(); reader.Options.CharacterSet = "UTF-8"; Result resultQR = reader.Decode(picture); if (resultQR != null) { textBox1.Text = "QR码:\r\n"; string[] strList = resultQR.Text.Split(','); for (int i = 0; i < strList.Length; i++) textBox1.AppendText(i.ToString("d2") + ":" + strList[i] + "\r\n"); timer1.Stop(); ShutCamera(); return; } } totalTime += delaytime; textBox1.Text = totalTime.ToString() + " ms"; //if (totalTime == 3000) // picture.Save("测试图片.bmp"); }解析代码意思
时间: 2024-02-15 14:27:56 浏览: 23
这是一个 C# 语言编写的 Windows 窗体程序中的计时器事件处理函数。该函数周期性地从视频源中获取当前帧图像,并使用 ZXing 库中的 BarcodeReader 类对该图像进行二维码的识别。如果识别到了二维码,就将其文本信息显示在 textBox1 控件中,并停止计时器和关闭摄像头。如果超过了最大识别时间(20秒),则也停止计时器和关闭摄像头,并在 textBox1 控件中显示一条未识别到二维码的提示信息。在处理期间,还会在 textBox1 控件中显示总共消耗的时间。注释中还有一段代码,是用于在 totalTime 达到 3000 毫秒时将当前帧图像保存为测试图片的代码,但是被注释掉了,不会执行。
相关问题
private void timer1_Tick(object sender, EventArgs e) { if (totalTime >= 20000)// 最多读20秒 { timer1.Stop(); ShutCamera(); textBox1.Text = "未识别到条形码"; return; } Bitmap barcodeImage; barcodeImage = videoSourcePlayer1.GetCurrentVideoFrame();//拍摄 if (barcodeImage != null) { // 识读条形码 BarcodeReader reader = new BarcodeReader(); reader.Options.CharacterSet = "UTF-8"; reader.Options.PossibleFormats = new List<BarcodeFormat>() { BarcodeFormat.CODE_128 }; Result resultBarcode = reader.Decode(barcodeImage); if (resultBarcode != null) { textBox1.Text = " "; textBox1.AppendText(resultBarcode.Text); timer1.Stop(); ShutCamera(); return; } } totalTime += delaytime; textBox1.Text = totalTime.ToString() + " ms"; //if (totalTime == 3000) // picture.Save("测试图片.bmp"); } private void button4_Click(object sender, EventArgs e) { string searchNum = textBox1.Text.Trim(); searchNum = searchNum.Substring(0, 8); // 只取前8位 string folderPath = textBox2.Text.Trim(); foreach (string filePath in Directory.GetFiles(folderPath, "*.pdf")) { string fileName = Path.GetFileNameWithoutExtension(filePath); if (fileName.Length >= 8) { string firstEightDigits = fileName.Substring(0, 8); if (firstEightDigits == searchNum) { Process.Start(filePath); return; // 打开第一个匹配的pdf文件后退出循环 } } } MessageBox.Show("未找到匹配的pdf文件。"); } 修改为扫描出条形码在文本框中只显示8位并打开第一个匹配的pdf文件后退出循环
好的,我理解你的问题了。请将代码修改为以下内容:
```
private void timer1_Tick(object sender, EventArgs e)
{
if (totalTime >= 20000)// 最多读20秒
{
timer1.Stop();
ShutCamera();
textBox1.Text = "未识别到条形码";
return;
}
Bitmap barcodeImage;
barcodeImage = videoSourcePlayer1.GetCurrentVideoFrame();//拍摄
if (barcodeImage != null)
{
// 识读条形码
BarcodeReader reader = new BarcodeReader();
reader.Options.CharacterSet = "UTF-8";
reader.Options.PossibleFormats = new List<BarcodeFormat>() { BarcodeFormat.CODE_128 };
Result resultBarcode = reader.Decode(barcodeImage);
if (resultBarcode != null)
{
textBox1.Text = " ";
string barcodeText = resultBarcode.Text.Length >= 8 ? resultBarcode.Text.Substring(0, 8) : resultBarcode.Text;
textBox1.AppendText(barcodeText);
timer1.Stop();
ShutCamera();
string folderPath = textBox2.Text.Trim();
foreach (string filePath in Directory.GetFiles(folderPath, "*.pdf"))
{
string fileName = Path.GetFileNameWithoutExtension(filePath);
if (fileName.Length >= 8)
{
string firstEightDigits = fileName.Substring(0, 8);
if (firstEightDigits == barcodeText)
{
Process.Start(filePath);
return; // 打开第一个匹配的pdf文件后退出循环
}
}
}
MessageBox.Show("未找到匹配的pdf文件。");
return;
}
}
totalTime += delaytime;
textBox1.Text = totalTime.ToString() + " ms";
//if (totalTime == 3000)
// picture.Save("测试图片.bmp");
}
```
修改后的代码会先判断识别到的条形码长度是否超过8位,如果超过则只取前8位,然后在文本框中显示。接着会遍历指定文件夹中的所有PDF文件,找到第一个文件名前8位与条形码匹配的文件并打开,然后退出循环。如果没有找到匹配的文件,则弹出提示框。
FilterInfoCollection videoDevices;//摄像头设备集合 VideoCaptureDevice videoSource;//捕获设备源 const int delaytime = 500; //扫码间隔时间 ms int totalTime; //扫码最长时间 public Form1() { InitializeComponent(); } private void comboBox1_SelectedIndexChanged(object sender, EventArgs e) { } private void button1_Click(object sender, EventArgs e) { comboBox1.Items.Clear(); videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice); for (int i = 0; i < videoDevices.Count; i++) comboBox1.Items.Add(videoDevices[i].Name); comboBox1.Text = comboBox1.Items[0].ToString(); } private void button2_Click(object sender, EventArgs e) { if (comboBox1.Text == null) return; videoSource = new VideoCaptureDevice(videoDevices[comboBox1.SelectedIndex].MonikerString); videoSourcePlayer1.VideoSource = videoSource; videoSourcePlayer1.Start(); textBox1.Text = "开始识读"; timer1.Interval = delaytime; timer1.Start(); totalTime = 0; } private void timer1_Tick(object sender, EventArgs e) { if (totalTime >= 20000)// 最多读20秒 { timer1.Stop(); textBox1.Text = "未识别到条形码"; return; } Bitmap barcodeImage; barcodeImage = videoSourcePlayer1.GetCurrentVideoFrame();//拍摄 if (barcodeImage != null) { // 识读条形码 BarcodeReader reader = new BarcodeReader(); reader.Options.CharacterSet = "UTF-8"; reader.Options.PossibleFormats = new List<BarcodeFormat>() { BarcodeFormat.CODE_128 }; Result resultBarcode = reader.Decode(barcodeImage); if (resultBarcode != null) { textBox1.Text = ""; textBox1.AppendText(resultBarcode.Text); timer1.Stop(); return; } } totalTime += delaytime; textBox1.Text = totalTime.ToString() + " ms"; //if (totalTime == 3000) // picture.Save("测试图片.bmp");怎么设置锁定后置摄像头不打开前置摄像头
要想实现锁定后置摄像头,不打开前置摄像头,可以在获取摄像头设备源时,指定后置摄像头的设备。具体实现方法如下:
1. 获取所有视频设备的集合
```
videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice);
```
2. 遍历视频设备集合,找到后置摄像头对应的设备
```
foreach (FilterInfo device in videoDevices)
{
if (device.Name == "后置摄像头名称")
{
videoSource = new VideoCaptureDevice(device.MonikerString);
break;
}
}
```
3. 如果找到后置摄像头对应的设备,则使用该设备进行视频捕捉,否则提示用户没有找到后置摄像头
```
if (videoSource != null)
{
videoSourcePlayer1.VideoSource = videoSource;
videoSourcePlayer1.Start();
}
else
{
MessageBox.Show("没有找到后置摄像头!");
}
```
需要注意的是,不同手机的后置摄像头名称可能不同,需要根据具体手机进行调试和修改。同时,如果找不到后置摄像头对应的设备,则需要在代码中处理异常情况。
相关推荐
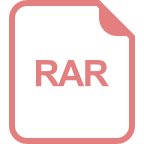
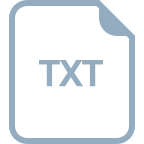
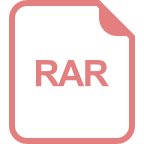







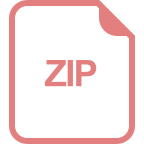