vue2 前端如何实现Markdown文档的导读功能,当用户点击导读列表中的某个链接时,页面将自动滚动到对应的标题处。
时间: 2024-02-17 21:04:40 浏览: 104
实现 Markdown 文档的导读功能,可以通过解析 Markdown 文档中的标题,生成一个导读列表,并通过锚点链接实现页面滚动到对应标题处的功能。具体实现步骤如下:
1. 安装依赖:`npm install markdown-it --save`
2. 引入 markdown-it:`import MarkdownIt from 'markdown-it'`
3. 创建 markdown-it 实例:`const md = new MarkdownIt()`
4. 解析 markdown 内容并生成 html:`const html = md.render(markdownContent)`
5. 使用正则表达式从生成的 html 中提取出所有标题(如 h1、h2、h3 等),并生成导读列表。
6. 将导读列表渲染到页面上,并为每个列表项添加锚点链接。
7. 监听锚点链接的点击事件,在点击时使用 JavaScript 实现页面滚动到对应标题处的功能。
以下是一个简单的实现示例:
```vue
<template>
<div>
<div class="markdown-body" v-html="html"></div>
<div class="toc">
<ul>
<li v-for="(item, index) in toc" :key="index">
<a :href="`#${item.id}`">{{ item.title }}</a>
</li>
</ul>
</div>
</div>
</template>
<script>
import MarkdownIt from 'markdown-it'
export default {
data() {
return {
html: '',
toc: [],
}
},
mounted() {
const md = new MarkdownIt()
const markdownContent = '# Title1\nContent1\n## Title2\nContent2\n### Title3\nContent3'
const html = md.render(markdownContent)
const toc = this.generateToc(html)
this.html = html
this.toc = toc
this.addScrollEvent()
},
methods: {
generateToc(html) {
const toc = []
const headers = html.match(/<h\d.*?>(.*?)<\/h\d>/gi)
if (headers) {
headers.forEach((header, index) => {
const level = header.match(/<h(\d).*?>/i)[1]
const title = header.replace(/<\/?h\d>/gi, '')
const id = `heading-${index}`
toc.push({ title, id, level })
})
}
return toc
},
addScrollEvent() {
const anchors = document.querySelectorAll('.toc a')
anchors.forEach((anchor) => {
anchor.addEventListener('click', (event) => {
event.preventDefault()
const target = document.getElementById(anchor.getAttribute('href').substring(1))
if (target) {
const targetTop = target.getBoundingClientRect().top + window.pageYOffset
window.scrollTo({ top: targetTop, behavior: 'smooth' })
}
})
})
},
},
}
</script>
```
在上面的示例中,我们首先创建了一个 markdown-it 实例,然后使用 render 方法将 markdown 内容转换成 html。接着,我们通过正则表达式从 html 中提取出所有标题,并生成导读列表。最后,将导读列表渲染到页面上,并为每个列表项添加锚点链接。我们还监听了锚点链接的点击事件,在点击时使用 JavaScript 实现页面滚动到对应标题处的功能。
需要注意的是,当页面滚动到对应标题处时,可能会因为标题栏的固定或其他原因导致滚动位置不准确。为了解决这个问题,可以在计算目标位置时加上一个偏移量,使滚动位置更准确。
阅读全文
相关推荐
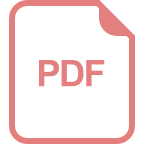
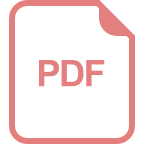
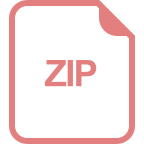
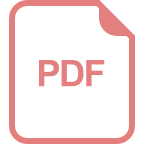
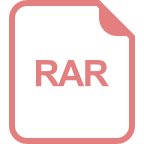
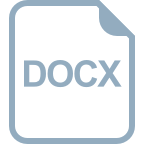
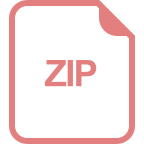
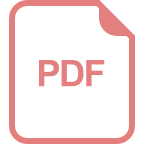
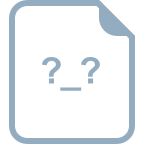
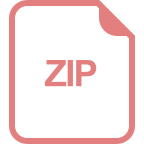
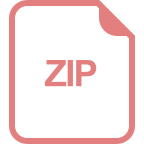
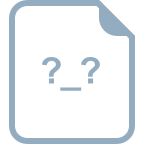
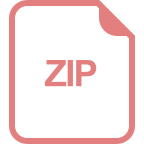