【程序练习】——每隔1秒向文件中写入一行记录 c语言
时间: 2023-08-20 18:04:07 浏览: 88
好的,这个问题我也可以回答。为了每隔1秒向文件中写入一行记录,我们可以使用C语言的time.h和stdio.h库。具体实现步骤如下:
1. 导入必要的库
```c
#include <time.h>
#include <stdio.h>
```
2. 定义文件指针和记录字符串
```c
FILE *fp;
char record[100];
```
3. 打开文件
```c
fp = fopen("record.txt", "a");
```
4. 循环写入记录
```c
while(1) {
time_t now = time(NULL); // 获取当前时间
strftime(record, sizeof(record), "%Y-%m-%d %H:%M:%S", localtime(&now)); // 格式化记录字符串
strcat(record, ": This is a record.\n"); // 拼接记录字符串
fputs(record, fp); // 写入文件
fflush(fp); // 刷新缓冲区
sleep(1); // 暂停1秒
}
```
5. 关闭文件
```c
fclose(fp);
```
这样,每隔1秒就会向文件中写入一行记录。注意,这个程序会一直执行下去,需要手动停止。
相关问题
【程序练习】——每隔1秒向文件中写入一行记录
好的,这个问题我可以回答。为了每隔1秒向文件中写入一行记录,我们可以使用Python中的time和datetime模块。具体实现步骤如下:
1. 导入time和datetime模块
```python
import time
import datetime
```
2. 打开文件
```python
file = open('record.txt', 'a')
```
3. 循环写入记录
```python
while True:
now = datetime.datetime.now() # 获取当前时间
record = f'{now}: This is a record.' # 构造记录字符串
file.write(record + '\n') # 写入文件
time.sleep(1) # 暂停1秒
```
4. 关闭文件
```python
file.close()
```
这样,每隔1秒就会向文件中写入一行记录。注意,这个程序会一直执行下去,需要手动停止。
编程读写一个文件,每隔一秒向文件中写入一行数据
下面是一个 Python 代码示例,可以每隔一秒向文件中写入一行数据:
```python
import time
filename = "test.txt"
with open(filename, "w") as f:
while True:
f.write("Hello, world!\n")
f.flush() # 立即将缓冲区的数据写入文件
time.sleep(1)
```
首先,我们使用 `open()` 函数打开一个文件,并指定文件模式为写入模式("w")。然后进入一个无限循环,每次循环向文件中写入一行数据,然后调用 `flush()` 方法将缓冲区的数据立即写入文件,最后通过 `time.sleep(1)` 实现每隔一秒写入一次数据。
注意,这个程序会一直运行下去,直到手动终止进程或发生异常。如果想要在一定时间后停止程序,可以在循环中添加一个计数器,并在达到一定次数后退出循环。
相关推荐
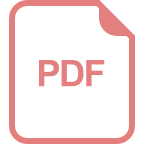












