linux c++ 复制文件的API是什么?
时间: 2024-11-22 22:42:05 浏览: 6
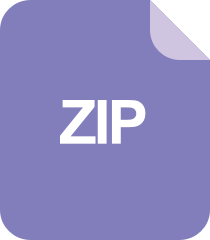
Linux命令 + C++API.zip
在Linux环境下,C++ API可以通过标准库函数来实现文件复制。以下是一个简单的示例,展示了如何使用`open()`、`read()`、`write()`和`close()`函数从源文件读取内容并写入目标文件:
```cpp
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#define BUF 32
int main(int argc, char** argv) {
if (argc != 3) {
// 检查参数数量
perror("Invalid arguments");
return -1;
}
int src_fd = open(argv[1], O_RDONLY); // 打开源文件
if (src_fd == -1) {
perror("Failed to open source file");
return -1;
}
int dst_fd = open(argv[2], O_WRONLY | O_CREAT, S_IRUSR | S_IWUSR); // 创建并打开目标文件
if (dst_fd == -1) {
perror("Failed to create destination file");
close(src_fd);
return -1;
}
int dup_fd = dup(src_fd); // 复制源文件描述符
if (dup_fd == -1) {
perror("Failed to duplicate file descriptor");
close(dst_fd);
close(src_fd);
return -1;
}
char buf[BUF];
ssize_t read_len;
while ((read_len = read(dup_fd, buf, BUF)) > 0) { // 逐块读取源文件内容
write(dst_fd, buf, read_len); // 写入目标文件
}
if (read_len == 0) { // 文件结束标志
printf("Source file ended.\n");
} else if (errno == EAGAIN || errno == EWOULDBLOCK) { // 可能是因为缓冲区已满
perror("Read error");
} else {
perror("Unknown read error");
}
close(src_fd); // 关闭源文件
close(dup_fd); // 关闭复制后的文件描述符
close(dst_fd); // 关闭目标文件
printf("File copied successfully.\n");
return 0;
}
```
这段代码接受两个参数,分别是源文件路径和目标文件路径,然后通过`open()`打开文件,`dup()`复制文件描述符,`read()`从源文件读取内容,`write()`写入目标文件,最后关闭所有文件句柄。
阅读全文
相关推荐
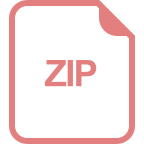
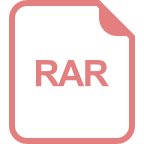

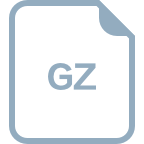
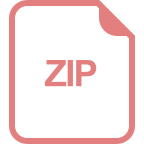
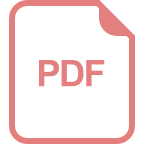
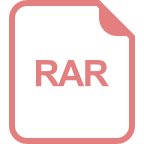
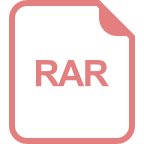
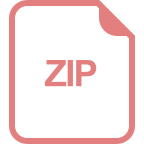
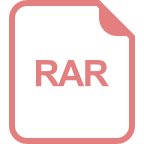
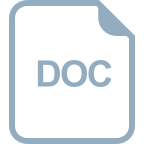
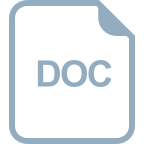
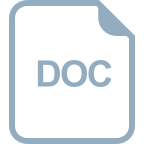
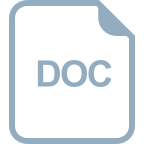
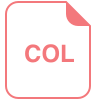
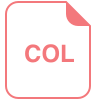


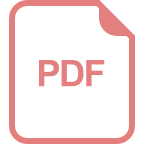