用platform bus driver的方式来写上述程序 ,给出设备树配置
时间: 2024-03-21 20:38:35 浏览: 14
好的,以下是使用platform bus driver的方式编写Winbond SPI Flash驱动程序的示例代码,以及相应的设备树配置。
首先,创建一个名为“winbond-spi-flash”或类似的设备节点,并将其添加到设备树中。以下是一个简单的设备树配置示例:
```dts
&spi0 {
winbond_spi_flash: winbond-spi-flash@0 {
compatible = "winbond,w25q64";
reg = <0>;
spi-max-frequency = <100000000>;
};
};
```
在驱动程序中,您需要实现probe和remove函数,用于初始化和清理设备资源。以下是一个简单的驱动程序示例:
```c
#include <linux/init.h>
#include <linux/module.h>
#include <linux/platform_device.h>
#include <linux/spi/spi.h>
#define WINBOND_CMD_READ_ID 0x9f
#define WINBOND_CMD_READ_DATA 0x03
static struct spi_device *winbond_spi_device;
static int winbond_spi_probe(struct platform_device *pdev)
{
int ret;
struct device *dev = &pdev->dev;
u8 id[3];
printk(KERN_INFO "winbond_spi: probe\n");
// get the SPI device pointer
winbond_spi_device = dev_get_drvdata(dev);
// read the Winbond Flash ID
struct spi_transfer transfer = {
.tx_buf = &WINBOND_CMD_READ_ID,
.rx_buf = id,
.len = 3,
};
struct spi_message message;
spi_message_init(&message);
spi_message_add_tail(&transfer, &message);
ret = spi_sync(winbond_spi_device, &message);
if (ret < 0) {
printk(KERN_ERR "winbond_spi: failed to read Winbond Flash ID\n");
return ret;
}
printk(KERN_INFO "winbond_spi: Winbond Flash ID: %02x %02x %02x\n",
id[0], id[1], id[2]);
return 0;
}
static int winbond_spi_remove(struct platform_device *pdev)
{
printk(KERN_INFO "winbond_spi: remove\n");
return 0;
}
static struct platform_driver winbond_spi_driver = {
.driver = {
.name = "winbond-spi-flash",
},
.probe = winbond_spi_probe,
.remove = winbond_spi_remove,
};
static int winbond_spi_probe(struct spi_device *spi)
{
return 0;
}
static int winbond_spi_remove(struct spi_device *spi)
{
return 0;
}
static struct spi_driver winbond_spi_driver = {
.driver = {
.name = "winbond-spi-flash",
},
.probe = winbond_spi_probe,
.remove = winbond_spi_remove,
};
static int __init winbond_spi_init(void)
{
int ret;
struct spi_master *master;
struct platform_device *pdev;
printk(KERN_INFO "winbond_spi: init\n");
// find the SPI master controller
master = spi_busnum_to_master(0);
if (!master) {
printk(KERN_ERR "winbond_spi: failed to find SPI master controller\n");
return -ENODEV;
}
// register the SPI driver
ret = spi_register_driver(&winbond_spi_driver);
if (ret < 0) {
printk(KERN_ERR "winbond_spi: failed to register SPI driver\n");
return ret;
}
// create the platform device
pdev = platform_device_alloc("winbond-spi-flash", 0);
if (!pdev) {
printk(KERN_ERR "winbond_spi: failed to allocate platform device\n");
return -ENOMEM;
}
platform_device_add(pdev);
return 0;
}
static void __exit winbond_spi_exit(void)
{
printk(KERN_INFO "winbond_spi: exit\n");
// remove the platform device
platform_device_unregister(&winbond_spi_device->dev);
// unregister the SPI driver
spi_unregister_driver(&winbond_spi_driver);
}
MODULE_LICENSE("GPL");
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("Winbond SPI Flash Driver");
module_init(winbond_spi_init);
module_exit(winbond_spi_exit);
```
在该驱动程序中,probe函数用于初始化SPI总线并读取Winbond Flash的ID。在remove函数中,您可以清理和释放设备资源。
需要注意的是,驱动程序中的设备树配置和平台驱动程序名称需要匹配。在本例中,设备树中的节点名称为“winbond-spi-flash”,而平台驱动程序的名称为“winbond-spi-flash”。
相关推荐
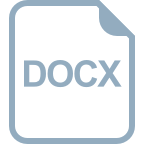
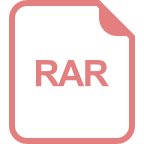














