使用Java写一份拼图游戏
时间: 2023-05-20 12:04:40 浏览: 82
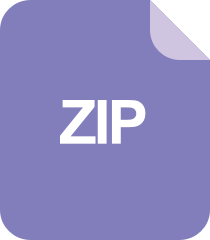
课程大作业-基于Java实现的拼图游戏源码+项目说明+详细设计.zip
好的,我可以为您提供一份使用Java编写的拼图游戏。以下是代码示例:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class PuzzleGame extends JFrame implements ActionListener {
private JPanel panel;
private JButton[] buttons;
private ImageIcon[] images;
private int[] puzzle;
private int emptyIndex;
public PuzzleGame() {
setTitle("拼图游戏");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 400);
setLocationRelativeTo(null);
panel = new JPanel(new GridLayout(3, 3));
add(panel, BorderLayout.CENTER);
images = new ImageIcon[9];
images[0] = new ImageIcon("image1.jpg");
images[1] = new ImageIcon("image2.jpg");
images[2] = new ImageIcon("image3.jpg");
images[3] = new ImageIcon("image4.jpg");
images[4] = new ImageIcon("image5.jpg");
images[5] = new ImageIcon("image6.jpg");
images[6] = new ImageIcon("image7.jpg");
images[7] = new ImageIcon("image8.jpg");
images[8] = new ImageIcon("image9.jpg");
puzzle = new int[9];
for (int i = 0; i < puzzle.length; i++) {
puzzle[i] = i;
}
emptyIndex = 8;
buttons = new JButton[9];
for (int i = 0; i < buttons.length; i++) {
buttons[i] = new JButton(images[puzzle[i]]);
buttons[i].addActionListener(this);
panel.add(buttons[i]);
}
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
int index = -1;
for (int i = 0; i < buttons.length; i++) {
if (e.getSource() == buttons[i]) {
index = i;
break;
}
}
if (index == emptyIndex - 1 || index == emptyIndex + 1 || index == emptyIndex - 3 || index == emptyIndex + 3) {
buttons[emptyIndex].setIcon(buttons[index].getIcon());
buttons[index].setIcon(images[8]);
puzzle[emptyIndex] = puzzle[index];
puzzle[index] = 8;
emptyIndex = index;
}
if (isComplete()) {
JOptionPane.showMessageDialog(this, "恭喜你完成了拼图!");
}
}
private boolean isComplete() {
for (int i = 0; i < puzzle.length; i++) {
if (puzzle[i] != i) {
return false;
}
}
return true;
}
public static void main(String[] args) {
new PuzzleGame();
}
}
```
这是一个简单的拼图游戏,使用了Java的Swing库来创建GUI界面。在这个游戏中,玩家需要通过点击拼图块来移动它们,直到拼图完成。
阅读全文
相关推荐
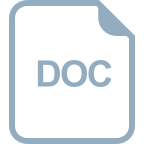
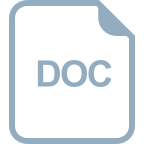
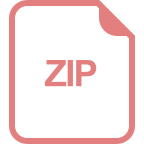
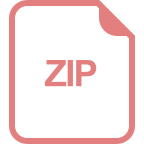
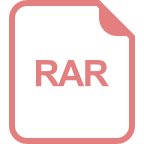
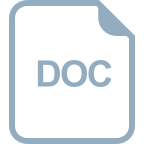
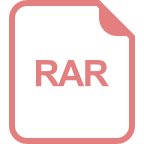
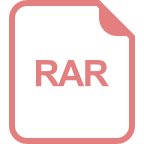
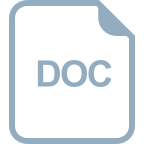
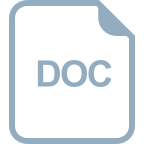
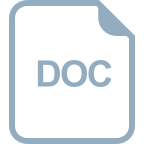
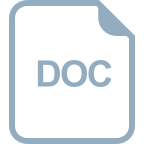
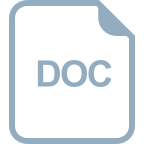
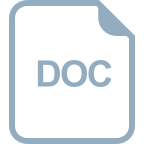
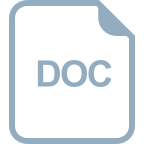


