std::unique_ptr<Json::CharReader> jsonReader{ readerBuilder.newCharReader()};
时间: 2024-05-19 14:12:32 浏览: 17
This line of code creates a new `std::unique_ptr` object named `jsonReader`, which is a smart pointer that owns and manages a dynamically allocated `Json::CharReader` object. The `newCharReader()` function is called on an instance of `Json::CharReaderBuilder`, which returns a pointer to a new `Json::CharReader` object. The `std::unique_ptr` takes ownership of this object and will automatically delete it when it goes out of scope.
相关问题
std::vector<std::unique_ptr<>>
std::vector<std::unique_ptr<>>是一种容器类型,它可以存储指向动态分配对象的智能指针。每个智能指针都负责管理其指向的对象的内存释放。下面是一个示例演示如何使用std::vector<std::unique_ptr<>>:
```cpp
#include <iostream>
#include <memory>
#include <vector>
class MyObject {
public:
MyObject(int value) : m_value(value) {
std::cout << "Constructing MyObject with value: " << m_value << std::endl;
}
~MyObject() {
std::cout << "Destructing MyObject with value: " << m_value << std::endl;
}
void printValue() {
std::cout << "Value: " << m_value << std::endl;
}
private:
int m_value;
};
int main() {
std::vector<std::unique_ptr<MyObject>> objects;
objects.push_back(std::make_unique<MyObject>(1));
objects.push_back(std::make_unique<MyObject>(2));
objects.push_back(std::make_unique<MyObject>(3));
for (const auto& obj : objects) {
obj->printValue();
}
return 0;
}
```
这个例子创建了一个std::vector<std::unique_ptr<MyObject>>,并向其中添加了三个MyObject对象。当vector离开作用域时,它会自动释放所有的MyObject对象。在循环中,我们打印了每个对象的值。
std::unique_ptr<std::thread> thread
std::unique_ptr<std::thread> thread是一个智能指针,用于管理std::thread类型的对象。它的定义是通过引用给出的重载 (6),它可以隐式转换为std::unique_ptr<Base>,因为被管理的指针和std::default_delete都可以隐式转换。这意味着std::unique_ptr<std::thread> thread可以自动释放所拥有的std::thread对象,并确保资源的正确清理。同时,std::unique_ptr<std::thread> thread还可以使用std::weak_ptr进行非拥有性引用。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [c++11 智能指针 (std::unique_ptr)(一)](https://blog.csdn.net/qq_40788199/article/details/126687120)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [c++11:智能指针之std::unique_ptr、std::shared_ptr、std::weak_ptr](https://blog.csdn.net/weixin_40179091/article/details/109757523)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
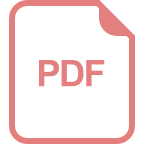












