关于spring boot面试题以及答案
时间: 2023-08-23 09:05:44 浏览: 178
以下是常见的 Spring Boot 面试题及其答案:
1. 什么是 Spring Boot?
Spring Boot 是基于 Spring 框架的快速开发脚手架,它提供了常用的配置和依赖管理,使得开发者可以快速构建独立的、生产级别的 Spring 应用程序,而不需要过多的配置。
2. Spring Boot 和 Spring 框架的区别是什么?
Spring Boot 是 Spring 框架的扩展,它提供了自动化配置、快速开发、无需 XML 配置文件等特性,使得开发者能够更快速地构建 Spring 应用程序。
3. Spring Boot 的优点有哪些?
Spring Boot 的优点有:
- 快速开发:Spring Boot 提供了自动化配置和依赖管理,使得开发者能够更快速地构建应用程序。
- 简化配置:Spring Boot 提供了默认配置,开发者可以根据需要进行修改,而无需进行繁琐的配置。
- 易于部署:Spring Boot 应用程序可以打包成 jar 包或 war 包进行部署,无需安装应用程序的容器。
- 微服务:Spring Boot 支持微服务开发,可以使用 Spring Cloud 进行服务注册、发现、路由等操作。
- 社区活跃:Spring Boot 拥有庞大的社区,开发者可以从中获取各种资料、解决问题。
4. Spring Boot 中的 starter 是什么?
Spring Boot 中的 starter 是一组预定义的依赖,它们包含了常用的类库或框架,开发者可以直接引入这些依赖,而无需手动配置相关的依赖。
5. Spring Boot 中的自动配置是什么?
Spring Boot 中的自动配置是一种特殊的配置,它会根据应用程序的 classpath 中的 jar 包和配置文件,自动配置应用程序的环境。开发者可以通过注解或配置文件进行覆盖或修改。
6. Spring Boot 如何实现热部署?
Spring Boot 可以通过添加 devtools 依赖实现热部署。在 pom.xml 中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<optional>true</optional>
<scope>runtime</scope>
</dependency>
```
7. Spring Boot 如何实现跨域访问?
Spring Boot 可以通过添加 cors 配置实现跨域访问。在配置类中添加以下代码:
```java
@Configuration
public class CorsConfig {
@Bean
public WebMvcConfigurer corsConfigurer() {
return new WebMvcConfigurer() {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*")
.allowedMethods("GET", "POST", "PUT", "DELETE")
.allowCredentials(false).maxAge(3600);
}
};
}
}
```
8. Spring Boot 如何实现缓存?
Spring Boot 可以通过添加缓存依赖实现缓存。在 pom.xml 中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
```
在配置类中添加以下代码:
```java
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("cacheName");
}
}
```
在需要缓存的方法上添加 @Cacheable 注解即可实现缓存。
9. Spring Boot 如何实现安全认证?
Spring Boot 可以通过添加 security 依赖实现安全认证。在 pom.xml 中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
在配置类中添加以下代码:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")
.antMatchers("/").permitAll()
.and()
.formLogin()
.and()
.logout()
.logoutUrl("/logout")
.logoutSuccessUrl("/login")
.invalidateHttpSession(true)
.deleteCookies("JSESSIONID");
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}password").roles("ADMIN");
}
}
```
在需要认证的方法上添加 @Secured 注解即可实现安全认证。
以上是常见的 Spring Boot 面试题及其答案,希望对你有所帮助。
阅读全文
相关推荐
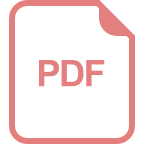
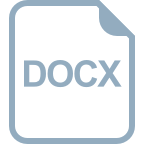
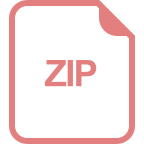
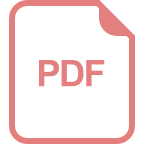
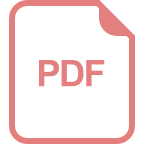
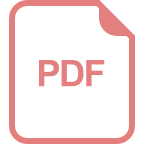
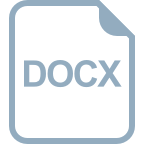




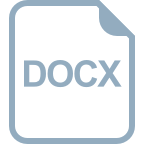
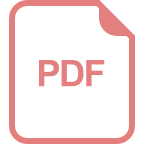
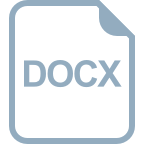
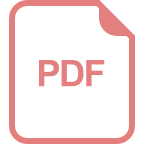